python截取wav音频
时间: 2023-10-29 08:54:08 浏览: 81
要实现Python截取WAV音频,你可以按照以下步骤进行操作:
1. 导入必要的库:使用Python的wave库来读取WAV文件。
2. 打开WAV文件:使用wave.open()函数打开指定的WAV文件。
3. 获取音频信息:通过读取WAV文件的getparams()方法来获取音频的参数信息,如采样频率、声道数等。
4. 设置截取的起止时分秒:根据需要,设置起止时分秒来确定要截取的音频段落。
5. 计算截取的帧数:根据音频的采样率和设置的起止时分秒,计算出对应的帧数范围。
6. 设置输出音频信息:根据需要,设置截取出来的音频的名称、专辑名称、歌手名称等信息。
7. 创建输出音频文件:使用wave.open()函数创建一个新的WAV文件来存储截取出来的音频。
8. 读取并写入截取的音频数据:使用WAV文件的readframes()方法读取指定范围的音频帧,并使用writeframes()方法将这些帧写入到新的WAV文件中。
9. 关闭文件:使用WAV文件的close()方法关闭输入和输出文件。
以下是一个示例代码,演示了如何使用Python截取WAV音频:
```python
import wave
# 打开WAV文件
input_file = wave.open("input.wav", "rb")
output_file = wave.open("output.wav", "wb")
# 获取音频信息
params = input_file.getparams()
# 设置截取的起止时分秒
start_time = 0 # 设置起始时间(秒)
end_time = 10 # 设置结束时间(秒)
# 计算截取的帧数
start_frame = int(start_time * params.framerate)
end_frame = int(end_time * params.framerate)
# 设置输出音频信息
output_file.setparams(params)
# 创建输出音频文件
output_file.writeframes(input_file.readframes(start_frame))
output_file.writeframes(input_file.readframes(end_frame - start_frame))
# 关闭文件
input_file.close()
output_file.close()
```
请注意,上述示例代码仅展示了截取WAV音频的基本步骤,你可以根据实际需求进行更进一步的处理和扩展。
相关推荐
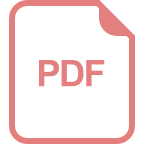












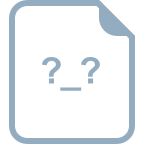
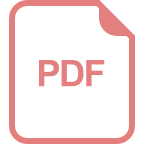