创建一个时钟窗体界面,包含时针,分针,秒针。随时间变化,秒针走的。以及窗体标题中时间显示。包含picturebox,label控件,Timer控件及其事件激发。时钟中数字需自行设置。c#全代码
时间: 2024-11-13 07:40:52 浏览: 50
创建一个简单的Windows Forms应用程序,展示一个带有时针、分针和秒针的计时器,以及实时更新的窗口标题,我们可以使用C#的System.Windows.Forms库。以下是基本的步骤和代码示例:
首先,我们需要添加所需控件到Form中:
```xml
<WindowsFormsApplication1>
<Form Name="Form1" Text="C# 自定义时钟" StartPosition="CenterScreen">
<PictureBox Name="pictureBox1" />
<Label Name="labelTime" Font="Arial, 40pt" AutoSize=False TextAlign="Center" />
</Form>
</WindowsFormsApplication1>
```
然后,在Form1.cs文件中编写代码:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
public partial class Form1 : Form
{
private Timer timer;
private int[] color = { Color.Black, Color.Gray }; // 针的颜色数组
private Random randomizer = new Random();
public Form1()
{
InitializeComponent();
// 设置初始时间和秒针位置
InitializeClock(0);
// 初始化定时器,每秒更新一次
timer = new Timer();
timer.Interval = 1000; // 1000毫秒等于1秒
timer.Tick += Timer_Tick;
timer.Start();
}
private void InitializeClock(int seconds)
{
labelTime.Text = FormatTime(seconds);
pictureBox1.Image = GetHandImage(TimeSpan.FromSeconds(seconds), color[randomizer.Next(color.Length)]);
}
private string FormatTime(int seconds)
{
int minutes = seconds / 60;
int hours = minutes / 60;
int minutesRemainder = minutes % 60;
int secondsRemainder = seconds % 60;
return $"{hours:D2}:{minutesRemainder:D2}:{secondsRemainder:D2}";
}
private Image GetHandImage(TimeSpan time, Color color)
{
double angle = (double)time.TotalSeconds * (Math.PI * 2) / 60; // 计算指针角度
Bitmap bitmap = new Bitmap(100, 100); // 画布大小
Graphics g = Graphics.FromImage(bitmap);
Pen pen = new Pen(color);
// 绘制时针、分针和秒针
DrawHand(g, pen, angle, 15, 85); // 15度,85长度(假设)
DrawHand(g, pen, angle + Math.PI / 6, 12, 75); // 分针
DrawHand(g, pen, angle + Math.PI / 30, 25, 40); // 秒针
return bitmap;
}
private static void DrawHand(Graphics g, Pen pen, double angle, int radius, int length)
{
g.RotateTransform(angle);
g.DrawLine(pen, 50, 50, 50 + length * Math.Cos(angle), 50 + length * Math.Sin(angle));
}
private void Timer_Tick(object sender, EventArgs e)
{
InitializeClock(timer.Interval);
}
}
```
这个例子中,我们创建了一个定时器,每秒触发`Timer_Tick`事件,更新计时器和标签。时钟的外观样式可以根据需要自定义。
阅读全文
相关推荐




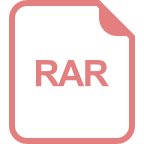




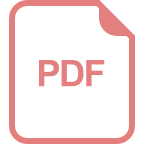
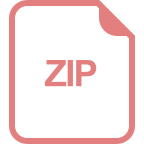
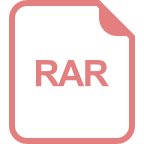
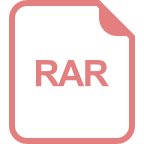

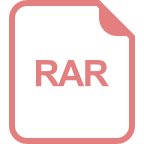
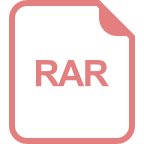


