用蚁群聚类算法对数据实现聚类处理的python实现
时间: 2024-04-21 22:22:14 浏览: 89
以下是使用蚁群聚类算法对数据进行聚类处理的Python实现示例:
```python
import numpy as np
# 数据准备
data = np.array([[1, 2], [1, 4], [2, 2], [2, 4], [4, 4], [4, 6], [5, 5], [6, 4], [6, 6], [7, 5]])
# 蚁群聚类参数设置
num_ants = 10 # 蚂蚁数量
num_clusters = 2 # 聚类簇数
num_iterations = 100 # 迭代次数
# 初始化蚁群位置和信息素矩阵
num_points = data.shape[0]
ant_positions = np.random.randint(num_clusters, size=(num_ants, num_points))
pheromone_matrix = np.ones((num_clusters, num_points))
# 迭代更新
for _ in range(num_iterations):
# 计算每只蚂蚁的适应度值(距离)
fitness_values = np.zeros(num_ants)
for ant in range(num_ants):
cluster_centers = np.zeros((num_clusters, data.shape[1]))
for cluster in range(num_clusters):
cluster_points = data[ant_positions[ant] == cluster]
cluster_centers[cluster] = np.mean(cluster_points, axis=0)
distances = np.linalg.norm(data - cluster_centers[ant_positions[ant]], axis=1)
fitness_values[ant] = np.sum(np.min(distances))
# 更新信息素矩阵
delta_pheromone = np.zeros((num_clusters, num_points))
for ant in range(num_ants):
for point in range(num_points):
delta_pheromone[ant_positions[ant, point], point] += 1 / fitness_values[ant]
pheromone_matrix += delta_pheromone
# 更新蚂蚁位置
for ant in range(num_ants):
for point in range(num_points):
probabilities = pheromone_matrix[:, point] / np.sum(pheromone_matrix[:, point])
ant_positions[ant, point] = np.random.choice(num_clusters, p=probabilities)
# 聚类结果可视化
import matplotlib.pyplot as plt
colors = ['r', 'g', 'b', 'c', 'm', 'y', 'k']
for i in range(num_points):
color = colors[ant_positions[0, i]]
plt.scatter(data[i, 0], data[i, 1], c=color)
plt.show()
```
这个示例演示了如何使用蚁群聚类算法将数据分为两个聚类簇。你可以根据自己的数据进行调整和修改。请注意,蚂蚁数量、聚类簇数和迭代次数等参数可以根据需要进行调整。此外,还可以根据需要对信息素更新和蚂蚁位置更新的方法进行修改。
阅读全文
相关推荐
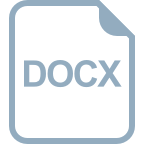
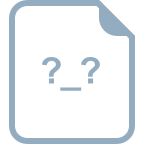
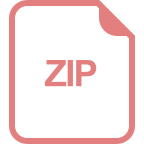
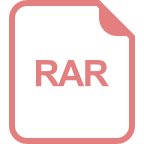
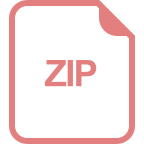
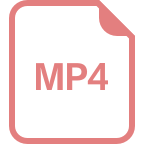
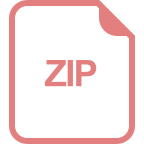