c语言自定义函数加载图片数据到buffer
时间: 2024-09-28 16:12:25 浏览: 32
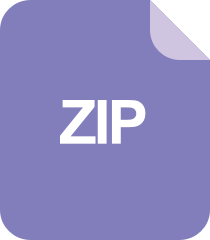
PostgreSQL简单函数创建

在C语言中,由于标准库并不直接提供处理图像的功能,你需要借助一些第三方库,比如`libpng`或者`stb_image`等。这里简单说明一个使用`stb_image`库加载图片数据到缓冲区的例子:
首先,你需要在你的工程中包含`stb_image.h`头文件,并链接相应的静态库(例如对于Windows使用`stb_image.lib`):
```c
#include "stb_image.h"
#include <stdint.h>
```
然后,你可以创建一个自定义函数来加载图片:
```c
typedef struct {
int width;
int height;
unsigned char* data;
} ImageData;
ImageData load_image(const char* filename) {
ImageData imageData;
const int success = stbi_load_from_file(&imageData.data, filename, &imageData.width, &imageData.height, nullptr, STBI_rgb_alpha);
if (!success) {
printf("Failed to load image %s\n", filename);
return imageData;
}
imageData.height *= -1; // Some libraries require a flipped Y-axis
imageData.width *= sizeof(unsigned char); // Calculate buffer size (assuming 32-bit RGB images)
imageData.data = (unsigned char*)malloc(imageData.width);
memcpy(imageData.data, imageData.data, imageData.width);
return imageData;
}
```
这个`load_image`函数接受一个文件名作为输入,尝试从文件加载PNG或JPG图像,将其转换为RGB颜色模式,并将数据存储到`ImageData`结构的`data`成员中。注意,加载完成后可能会释放原始的数据指针,所以在使用之前最好复制一份到你的缓冲区中。
阅读全文
相关推荐
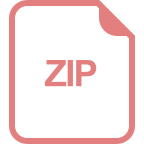
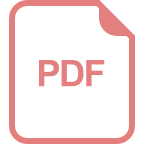
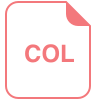
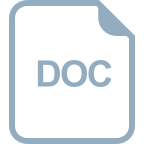
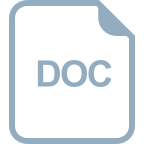
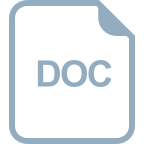
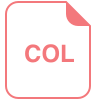
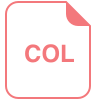
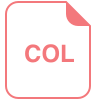
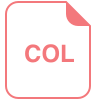
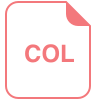
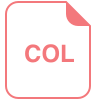
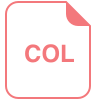
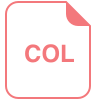
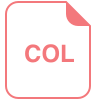
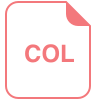
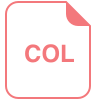
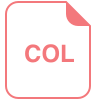