kotlin distinct
时间: 2023-12-22 18:00:09 浏览: 25
在 Kotlin 中,你可以使用 `distinct` 函数来获取一个列表中的不重复元素。这个函数会返回一个新的列表,其中只包含原始列表中的唯一元素。
```kotlin
val list = listOf(1, 2, 2, 3, 4, 4, 5)
val distinctList = list.distinct()
println(distinctList) // 输出 [1, 2, 3, 4, 5]
```
在上面的例子中,`list` 是一个包含重复元素的列表。通过调用 `distinct` 函数,我们得到了一个只包含不重复元素的列表 `distinctList`。最后的输出结果是 `[1, 2, 3, 4, 5]`。
相关问题
serialization kotlin
在Kotlin中,序列化是指将对象转换为字节流的过程,以便可以在网络上传输或者保存到文件中。Kotlin提供了一个内置的序列化框架,即Kotlin Serialization。下面是关于Kotlin Serialization的介绍:
1. Kotlin Serialization是什么?
Kotlin Serialization是Kotlin官方提供的一种轻量级的序列化框架,用于将Kotlin对象转换为字节流,以便进行传输或持久化存储。
2. 如何使用Kotlin Serialization?
使用Kotlin Serialization,你需要在你的Kotlin类上添加`@Serializable`注解,并且在属性上添加`@SerialName`注解来指定序列化后的字段名。然后,你可以使用`Json.encodeToString()`方法将对象序列化为JSON字符串,或使用`Json.decodeFromString()`方法将JSON字符串反序列化为对象。
3. Kotlin Serialization的特性有哪些?
Kotlin Serialization支持以下特性:
- 支持枚举类的序列化和反序列化
- 支持泛型类型的序列化和反序列化
kotlin Unit
Kotlin是一种基于JVM的静态类型编程语言,旨在成为Java的改进版。Unit是Kotlin中的一种类型,它表示一个不返回任何有用值的函数或表达式。在Java中,类似的概念是void。与Java不同的是,Kotlin中的Unit是一个真正的对象类型,而不是Java中的关键字。
在Kotlin中,函数可以返回Unit类型来表示其没有返回值。例如,下面的函数就返回了Unit类型:
fun printMessage(message: String): Unit {
println(message)
}
与Java不同的是,Kotlin中的Unit类型可以省略。如果函数确实没有返回值,则可以将其声明为不返回任何内容,如下所示:
fun printMessage(message: String) {
println(message)
}
这两个函数的行为是相同的。如果您调用它们,它们都会打印出传递给它们的消息字符串,并且不返回任何值。
相关推荐
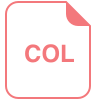











