用winform自定义一个边框颜色为黑白阴影的矩形可指定宽度的窗体控件
时间: 2024-09-20 12:09:13 浏览: 31
在Windows Form应用程序中,如果你想要自定义一个带有黑白阴影边缘效果的矩形控件,可以创建一个新的用户控件(UserControl)。以下是一个简单的步骤:
1. **新建UserControl**:
- 在Visual Studio中,右键点击项目 -> 添加 -> 新项 -> UserControl。
- 设计界面时,使用Graphics API绘制矩形并添加阴影效果。
```csharp
public partial class CustomRectangle : UserControl
{
public CustomRectangle()
{
InitializeComponent();
// 创建画布
var graphics = CreateGraphics();
var pen = new Pen(Color.Black, 2); // 黑色线条
// 绘制矩形
using (pen)
graphics.DrawRectangle(pen, ClientRectangle);
// 绘制白色内阴影
DrawInnerShadow(graphics);
graphics.Dispose();
}
private void DrawInnerShadow(Graphics g)
{
int dx = 1, dy = 1; // 阴影偏移量
float radius = 4f; // 阴影半径
Color color = Color.White * 0.7f; // 白色阴影颜色
for (int i = 0; i <= dx; i++)
{
int left = ClientRectangle.Left + i;
int top = ClientRectangle.Top;
int right = ClientRectangle.Right - i;
int bottom = ClientRectangle.Bottom;
using (var shadowPen = new Pen(color, 1))
g.DrawLine(shadowPen, left, top, right, top);
using (var shadowPen = new Pen(color, 1))
g.DrawLine(shadowPen, right, top, right, bottom);
using (var shadowPen = new Pen(color, 1))
g.DrawLine(shadowPen, right, bottom, left, bottom);
using (var shadowPen = new Pen(color, 1))
g.DrawLine(shadowPen, left, bottom, left, top);
if (i > 0)
{
using (var shadowPen = new Pen(color, 2))
g.DrawLine(shadowPen, left + radius, top, right - radius, top);
using (var shadowPen = new Pen(color, 2))
g.DrawLine(shadowPen, right - radius, top, right - radius, bottom);
using (var shadowPen = new Pen(color, 2))
g.DrawLine(shadowPen, left + radius, bottom, left + radius, top);
}
}
for (int i = 0; i <= dy; i++)
{
int left = ClientRectangle.Left;
int top = ClientRectangle.Top + i;
int right = ClientRectangle.Right;
int bottom = ClientRectangle.Bottom - i;
using (var shadowPen = new Pen(color, 1))
g.DrawLine(shadowPen, left, top, left, bottom);
using (var shadowPen = new Pen(color, 1))
g.DrawLine(shadowPen, right, top, right, bottom);
using (var shadowPen = new Pen(color, 1))
g.DrawLine(shadowPen, right, bottom, left, bottom);
using (var shadowPen = new Pen(color, 1))
g.DrawLine(shadowPen, left, bottom, left, top);
if (i > 0)
{
using (var shadowPen = new Pen(color, 2))
g.DrawLine(shadowPen, left, top + radius, left, bottom - radius);
using (var shadowPen = new Pen(color, 2))
g.DrawLine(shadowPen, right, top + radius, right, bottom - radius);
using (var shadowPen = new Pen(color, 2))
g.DrawLine(shadowPen, left, top + radius, right, top + radius);
}
}
}
}
```
现在你可以将这个自定义控件添加到任何Form中,并通过`Width`和`Height`属性指定其大小。记得在自定义控件的设计视图里设置适当的尺寸和约束,以便用户能够轻松调整。
阅读全文
相关推荐
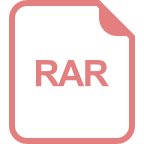
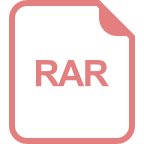
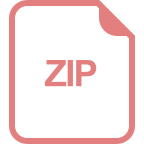
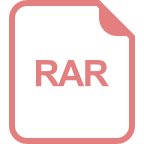
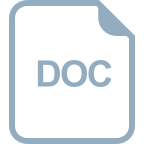



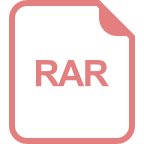
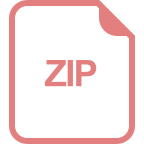
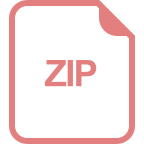
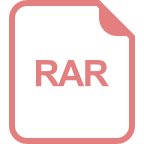
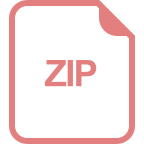
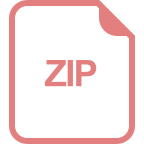