定义一个整形数组,将自己学号后10位同学的学号存入数组用for循环实现直接在程序中赋值将其逆序输出用for循环实现,将第5个数跟第9个数交换用数组实现,第六个数与第2数交换用指针实现c++
时间: 2024-09-06 14:06:56 浏览: 30
定义一个整型数组并将学号存入,然后逆序输出,以及交换特定位置的元素,可以通过以下C++代码实现:
```cpp
#include <iostream>
using namespace std;
int main() {
// 假设学号后10位为 1234567890,这里只是示例,应替换为实际的学号
int studentIDs[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
// 输出原始数组
cout << "原始数组: ";
for (int i = 0; i < 10; i++) {
cout << studentIDs[i] << " ";
}
cout << endl;
// 逆序输出数组
cout << "逆序输出: ";
for (int i = 9; i >= 0; i--) {
cout << studentIDs[i] << " ";
}
cout << endl;
// 交换第5个数和第9个数
swap(studentIDs[4], studentIDs[8]);
cout << "交换第5个数和第9个数后的数组: ";
for (int i = 0; i < 10; i++) {
cout << studentIDs[i] << " ";
}
cout << endl;
// 交换第六个数和第二个数,使用指针
int* sixth = &studentIDs[5]; // 第六个数的指针
int* second = &studentIDs[1]; // 第二个数的指针
int temp = *sixth;
*sixth = *second;
*second = temp;
// 输出交换后的数组
cout << "交换第六个数和第二个数后的数组: ";
for (int i = 0; i < 10; i++) {
cout << studentIDs[i] << " ";
}
cout << endl;
return 0;
}
```
这段代码首先定义了一个包含10个整数的数组`studentIDs`,并假设了学号后10位(这里使用简单的连续数字作为示例)。接着,使用`for`循环以正常顺序和逆序输出数组内容。然后,使用`swap`函数交换了数组中第5个元素(索引为4)和第9个元素(索引为8)的位置。最后,通过指针直接交换了第六个元素和第二个元素的值,并输出最终的数组。
相关推荐
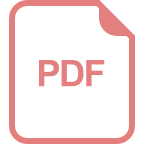
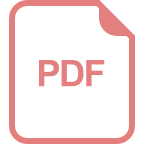
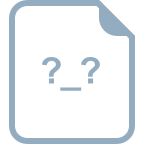















