@webservice注解配置 wsdl地址
时间: 2023-07-25 21:04:09 浏览: 143
你可以使用 `@WebService` 注解来配置 WSDL 地址。在注解中,你需要指定 `wsdlLocation` 属性来指定 WSDL 文件的地址。以下是一个示例:
```java
import javax.jws.WebService;
@WebService(wsdlLocation = "http://example.com/your_wsdl_file.wsdl")
public class YourWebService {
// ...
}
```
在上面的示例中,`wsdlLocation` 属性指定了 WSDL 文件的 URL 地址。你需要将其替换为你实际的 WSDL 文件的地址。这样配置后,你的 Web 服务将根据指定的 WSDL 文件进行发布和访问。
相关问题
spring环境中,使用@WebService开发接口并可供访问
可以按照以下步骤使用@WebService开发接口并可供访问:
1. 添加依赖:在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
```
2. 创建接口:创建一个接口并添加@WebService注解。例如:
```java
@WebService
public interface HelloWorld {
String sayHello(String name);
}
```
3. 实现接口:创建一个实现类并实现接口中的方法。例如:
```java
@Service
public class HelloWorldImpl implements HelloWorld {
@Override
public String sayHello(String name) {
return "Hello " + name + "!";
}
}
```
4. 配置WebService:在application.properties文件中添加以下配置:
```properties
# 指定WebService接口所在的包路径
spring.webservices.type-mapping-package=com.example.webservice
# 指定WebService服务的名称和命名空间
spring.webservices.namespace=http://example.com/webservice
spring.webservices.servlet.init.wsdl-namespace=http://example.com/webservice
spring.webservices.servlet.init.wsdl-location=classpath:/wsdl/helloworld.wsdl
```
5. 创建WSDL文件:在resources目录下创建wsdl文件夹,并在其中创建helloworld.wsdl文件。例如:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<wsdl:definitions xmlns:wsdl="http://schemas.xmlsoap.org/wsdl/" xmlns:tns="http://example.com/webservice" xmlns:xsd="http://www.w3.org/2001/XMLSchema" name="HelloWorld" targetNamespace="http://example.com/webservice">
<wsdl:types>
<xsd:schema targetNamespace="http://example.com/webservice">
<xsd:element name="sayHello">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="name" type="xsd:string"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
<xsd:element name="sayHelloResponse">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="return" type="xsd:string"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
</xsd:schema>
</wsdl:types>
<wsdl:message name="sayHelloRequest">
<wsdl:part element="tns:sayHello" name="parameters"/>
</wsdl:message>
<wsdl:message name="sayHelloResponse">
<wsdl:part element="tns:sayHelloResponse" name="parameters"/>
</wsdl:message>
<wsdl:portType name="HelloWorld">
<wsdl:operation name="sayHello">
<wsdl:input message="tns:sayHelloRequest"/>
<wsdl:output message="tns:sayHelloResponse"/>
</wsdl:operation>
</wsdl:portType>
<wsdl:binding name="HelloWorldSoap11" type="tns:HelloWorld">
<soap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<wsdl:operation name="sayHello">
<soap:operation soapAction=""/>
<wsdl:input>
<soap:body use="literal"/>
</wsdl:input>
<wsdl:output>
<soap:body use="literal"/>
</wsdl:output>
</wsdl:operation>
</wsdl:binding>
<wsdl:service name="HelloWorld">
<wsdl:port binding="tns:HelloWorldSoap11" name="HelloWorldSoap11">
<soap:address location="http://localhost:8080/ws"/>
</wsdl:port>
</wsdl:service>
</wsdl:definitions>
```
6. 启动应用程序:运行Spring Boot应用程序并访问http://localhost:8080/ws/helloworld.wsdl以获取WSDL信息。然后可以使用SOAP客户端或浏览器访问WebService接口。例如:
```xml
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:web="http://example.com/webservice">
<soapenv:Header/>
<soapenv:Body>
<web:sayHello>
<name>World</name>
</web:sayHello>
</soapenv:Body>
</soapenv:Envelope>
```
阅读全文
相关推荐
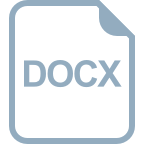
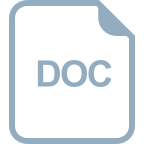
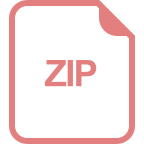
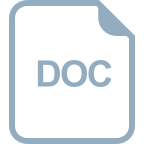
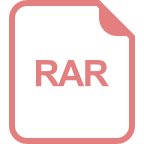
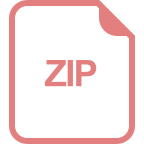
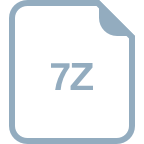
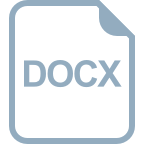
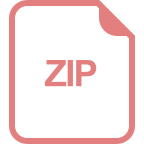
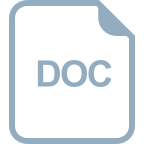
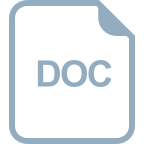