kotlin retrofit2 okhttp3
时间: 2024-12-26 12:20:04 浏览: 5
### Kotlin 中使用 Retrofit2 和 OkHttp3 进行网络请求
#### 添加依赖项
为了在项目中集成 Retrofit2 和 OkHttp3,需在 `build.gradle` 文件内加入必要的库:
```gradle
dependencies {
api 'com.squareup.okhttp3:logging-interceptor:3.6.0'
api 'com.squareup.retrofit2:retrofit:2.6.0'
api 'com.squareup.retrofit2:converter-gson:2.6.0'
}
```
这些依赖涵盖了 HTTP 客户端 (OkHttp),日志拦截器用于调试目的,RESTful API 工具包 (Retrofit) 及其 JSON 解析转换工具 Gson [^1]。
#### 构建 Retrofit 实例
创建一个名为 `ServiceCreator` 的单例类来初始化并返回 Retrofit 对象实例。此对象负责构建服务接口的具体实现版本,并提供访问远程服务器的能力。
```kotlin
object ServiceCreator {
private const val BASE_URL = "https://api.example.com/" // 替换成实际的基础URL
private val retrofit = Retrofit.Builder()
.baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.client(okHttpClient()) // 配置自定义 OkHttpClient 如果有特殊需求的话
.build()
fun <T> create(serviceClass: Class<T>): T = retrofit.create(serviceClass)
private fun okHttpClient(): OkHttpClient {
return OkHttpClient.Builder()
.addInterceptor(HttpLoggingInterceptor().setLevel(HttpLoggingInterceptor.Level.BODY))
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.writeTimeout(10, TimeUnit.SECONDS)
.build()
}
}
```
上述代码片段展示了如何通过设置基础 URL、添加 GSON 转换工厂以及配置带有日志记录功能的 OkHttpClient 来建立 Retrofit 实例 [^2]。
#### 定义 API 接口
接着定义与 RESTful Web Services 交互的服务接口。下面是一个简单的例子展示 GET 请求获取首页数据的方式:
```kotlin
interface ApiService {
@GET("/xxx/v1/homepage")
suspend fun getHomeData(@Query("ID") id: String): Response<HomeResponseData>
@FormUrlEncoded
@POST("/xxx/v1/homepage")
suspend fun postHomeData(
@Field("ID") id: String,
@Field("otherParam") otherParam: String
): Response<BaseResponseData<HomeResponseData>>
}
```
注意这里的函数签名采用了挂起修饰符 (`suspend`) 表明它们可以在协程环境中被调用 [^3]。
#### 发送请求
最后一步是在应用程序逻辑中利用前面准备好的组件发起具体的网络操作。通常情况下会封装在一个 Repository 或者 ViewModel 类里面执行异步任务。
```kotlin
class HomeRepository(private val apiService: ApiService) {
suspend fun fetchHomePage(id: String): Result<HomeResponseData> {
try {
val response = apiService.getHomeData(id)
if (response.isSuccessful && response.body() != null) {
return Result.success(response.body()!!)
} else {
throw Exception("Request failed with code ${response.code()}")
}
} catch (e: Exception) {
return Result.failure(e)
}
}
suspend fun submitFormData(id: String, param: String): Result<BaseResponseData<HomeResponseData>> {
try {
val response = apiService.postHomeData(id, param)
if (response.isSuccessful && response.body() != null) {
return Result.success(response.body()!!)
} else {
throw Exception("Submit request failed with code ${response.code()}")
}
} catch (e: Exception) {
return Result.failure(e)
}
}
}
```
这段代码实现了两个主要的功能——从服务器拉取主页信息和提交表单数据给服务器 [^5]。
阅读全文
相关推荐
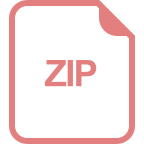
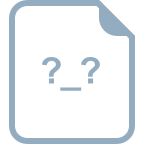
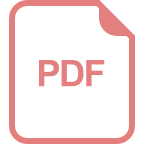
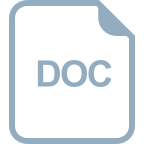
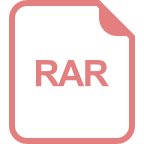
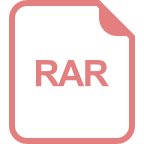
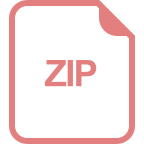
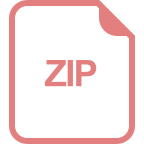
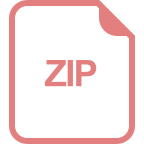
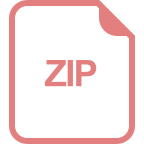
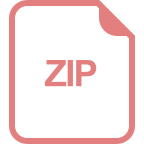
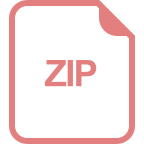
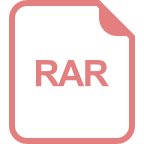
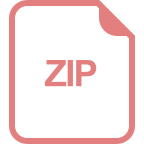
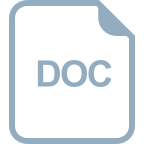



