如何在C语言中将用户输入的小写字母转换为大写字母?
时间: 2024-10-25 08:09:35 浏览: 26
在C语言中,你可以使用`toupper()`函数来将小写字母转换为大写字母。这个函数定义在`<ctype.h>`头文件里。其基本语法如下:
```c
#include <ctype.h>
char upper_case_char = toupper(original_char);
```
在这里,`original_char`是你想转换的小写字母,`upper_case_char`会得到转换后的对应的大写字母。注意,`toupper()`只对字母字符起作用,对于非字母字符(如数字、标点等),它不会改变。
如果你需要处理整个字符串,可以遍历字符串并逐个字符应用`toupper()`,例如:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
void to_uppercase(char *str) {
int i;
for (i = 0; str[i] != '\0'; i++) {
str[i] = toupper(str[i]);
}
}
int main() {
char input[50];
printf("Enter a string in lowercase: ");
fgets(input, sizeof(input), stdin); // Don't forget to remove newline at the end
to_uppercase(input);
printf("Uppercased string: %s\n", input);
return 0;
}
```
在这个示例中,`to_uppercase`函数会把输入字符串中的所有小写字母转换成大写。
阅读全文
相关推荐
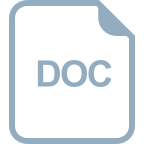
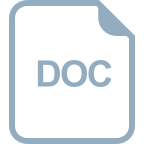
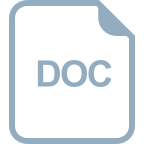
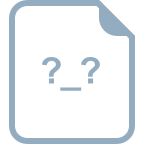
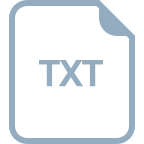





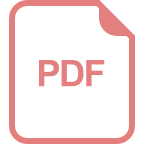
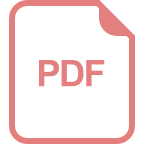






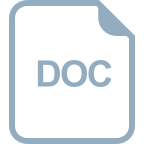