请再帮我指导一下圆形识别后如果有多个圆形,获取圆形的位置,并对每个圆形提取RGB值该如何写
时间: 2024-04-18 17:33:44 浏览: 136
在Halcon中,可以使用`FindCircle`函数进行圆形识别,然后通过`GetImagePointer3`函数获取图像的RGB值。以下是一个示例代码,展示了如何获取多个圆形的位置和对每个圆形提取RGB值:
```csharp
using HalconDotNet;
class Program
{
static void Main(string[] args)
{
// 读取图像
HImage image = new HImage("path_to_image");
// 转换为灰度图像
HImage grayImage = image.Rgb1ToGray();
// 边缘检测
HRegion edges = grayImage.EdgesSubPix("canny", 50, 80, 3);
// 查找圆形
HTuple rows, columns, radius;
HOperatorSet.FindCircle(edges, out rows, out columns, out radius);
// 获取图像的RGB数据
HTuple pointer, type, width, height;
HOperatorSet.GetImagePointer3(image, out pointer, out type, out width, out height);
byte[] imageData = pointer.ToDArr().ToByteArray();
// 遍历每个圆形
for (int i = 0; i < rows.Length; i++)
{
double row = rows[i].D;
double column = columns[i].D;
double circleRadius = radius[i].D;
// 提取圆形位置
Console.WriteLine($"Circle {i+1}: Row={row}, Column={column}, Radius={circleRadius}");
// 提取圆形RGB值
int centerX = (int)row;
int centerY = (int)column;
int circleSize = (int)circleRadius;
for (int x = centerX - circleSize; x <= centerX + circleSize; x++)
{
for (int y = centerY - circleSize; y <= centerY + circleSize; y++)
{
int index = (x * (int)width + y) * 3;
byte red = imageData[index];
byte green = imageData[index + 1];
byte blue = imageData[index + 2];
// 输出RGB值
Console.WriteLine($"Pixel at ({x},{y}): R={red}, G={green}, B={blue}");
}
}
}
// 释放资源
grayImage.Dispose();
edges.Dispose();
image.Dispose();
}
}
```
在上述代码中,我们首先通过`FindCircle`函数获取到多个圆形的位置信息,然后使用`GetImagePointer3`函数获取图像的RGB数据。接下来,我们遍历每个圆形,根据圆心和半径提取圆形的RGB值,并将其输出到控制台。
请注意,你需要将`"path_to_image"`替换为你实际的图像路径,并根据实际情况进行参数调整。同时,记得在使用完Halcon对象后,释放相应的资源。
阅读全文
相关推荐
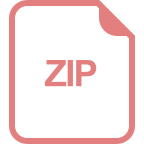
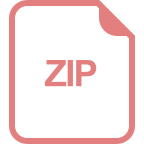
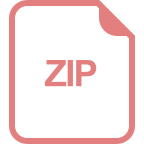
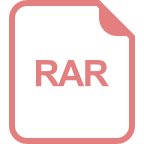
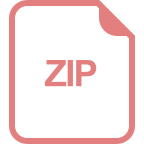