C# 指示灯控件使用
时间: 2023-11-23 22:57:37 浏览: 120
以下是使用C#自定义指示灯控件的步骤:
1. 首先,你需要在Visual Studio中创建一个新的WinForm项目。
2. 在解决方案资源管理器中,右键单击项目名称,然后选择“添加”>“用户控件”。
3. 在“添加新项”对话框中,选择“用户控件”模板,将名称设置为“IndicatorLightControl”,然后单击“添加”。
4. 在“IndicatorLightControl.cs”文件中,添加以下代码:
```csharp
public partial class IndicatorLightControl : UserControl
{
private bool _isBlinking = false;
private bool _isOn = false;
private Color _onColor = Color.Green;
private Color _offColor = Color.Red;
private int _blinkInterval = 500;
public IndicatorLightControl()
{
InitializeComponent();
}
public bool IsBlinking
{
get { return _isBlinking; }
set
{
_isBlinking = value;
if (_isBlinking)
{
blinkTimer.Start();
}
else
{
blinkTimer.Stop();
_isOn = false;
this.BackColor = _offColor;
}
}
}
public bool IsOn
{
get { return _isOn; }
set
{
_isOn = value;
if (_isOn)
{
this.BackColor = _onColor;
}
else
{
this.BackColor = _offColor;
}
}
}
public Color OnColor
{
get { return _onColor; }
set
{
_onColor = value;
if (_isOn)
{
this.BackColor = _onColor;
}
}
}
public Color OffColor
{
get { return _offColor; }
set
{
_offColor = value;
if (!_isOn)
{
this.BackColor = _offColor;
}
}
}
public int BlinkInterval
{
get { return _blinkInterval; }
set
{
_blinkInterval = value;
blinkTimer.Interval = _blinkInterval;
}
}
private void blinkTimer_Tick(object sender, EventArgs e)
{
_isOn = !_isOn;
if (_isOn)
{
this.BackColor = _onColor;
}
else
{
this.BackColor = _offColor;
}
}
}
```
5. 在“IndicatorLightControl.Designer.cs”文件中,添加以下代码:
```csharp
partial class IndicatorLightControl
{
private System.ComponentModel.IContainer components = null;
private System.Windows.Forms.Timer blinkTimer;
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.blinkTimer = new System.Windows.Forms.Timer(this.components);
this.SuspendLayout();
//
// blinkTimer
//
this.blinkTimer.Tick += new System.EventHandler(this.blinkTimer_Tick);
//
// IndicatorLightControl
//
this.BackColor = System.Drawing.Color.Red;
this.Name = "IndicatorLightControl";
this.Size = new System.Drawing.Size(20, 20);
this.ResumeLayout(false);
}
}
```
6. 在你的WinForm中,将“IndicatorLightControl”控件拖放到你的窗体上。
7. 在属性窗口中,你可以设置控件的颜色、是否闪烁以及闪烁速度等属性。
相关推荐
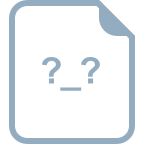














