Python绘画足球
时间: 2023-12-05 21:40:29 浏览: 31
以下是使用Python Matplotlib绘制足球的设计思路和实现效果:
1.设计思路:
- 创建一个圆形,表示足球。
- 在圆形中心绘制一个小圆,表示足球的中心点。
- 绘制两条直线,表示足球场的中线和中圈。
- 绘制四条弧线,表示足球场的四个角落。
- 绘制两个矩形,表示足球场的两个球门。
2.实现效果:
```python
import matplotlib.pyplot as plt
from matplotlib.patches import Arc, Circle, Rectangle
# 创建一个圆形,表示足球
def create_circle():
circle = Circle((0, 0), radius=9.15, color='black', fill=False)
return circle
# 在圆形中心绘制一个小圆,表示足球的中心点
def create_center_circle():
center_circle = Circle((0, 0), radius=0.4, color='black', fill=True)
return center_circle
# 绘制两条直线,表示足球场的中线和中圈
def create_lines():
lines = [plt.plot([0, 0], [i, -i], color='white', linewidth=2, zorder=5)[0] for i in range(40)]
lines += [plt.plot([i, -i], [0, 0], color='white', linewidth=2, zorder=5)[0] for i in range(60)]
center_circle = Circle((0, 0), radius=9.15, color='white', fill=False, linewidth=2, zorder=5)
lines.append(center_circle)
return lines
# 绘制四条弧线,表示足球场的四个角落
def create_corners():
corners = [Arc((55, 68), width=18.3, height=18.3, angle=0, theta1=270, theta2=360, color='white', linewidth=2, zorder=5),
Arc((55, 0), width=18.3, height=18.3, angle=0, theta1=0, theta2=90, color='white', linewidth=2, zorder=5),
Arc((-55, 68), width=18.3, height=18.3, angle=0, theta1=180, theta2=270, color='white', linewidth=2, zorder=5),
Arc((-55, 0), width=18.3, height=18.3, angle=0, theta1=90, theta2=180, color='white', linewidth=2, zorder=5)]
return corners
# 绘制两个矩形,表示足球场的两个球门
def create_goals():
goals = [Rectangle((-55, 25), 2.5, 18.3, color='white', fill=False, linewidth=2, zorder=5),
Rectangle((52.5, 25), 2.5, 18.3, color='white', fill=False, linewidth=2, zorder=5)]
return goals
# 绘制足球场
def create_soccer_field():
fig, ax = plt.subplots(figsize=(10.5, 6.8))
ax.set_xlim(-60, 60)
ax.set_ylim(-5, 73)
ax.axis('off')
ax.add_patch(create_circle())
ax.add_patch(create_center_circle())
ax.add_patch(create_goals()[0])
ax.add_patch(create_goals()[1])
for line in create_lines():
ax.add_line(line)
for corner in create_corners():
ax.add_patch(corner)
plt.show()
create_soccer_field()
```
相关推荐
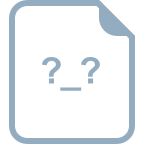














