c++ 截取HTML字符串中的内容
时间: 2024-09-15 09:05:26 浏览: 77
在C++中,截取HTML字符串中的特定内容通常需要先解析HTML,因为标准库并不直接提供这样的功能。可以借助第三方库如pugixml、htmlcxx或者更专业的库如BeautifulSoup(虽然这通常是Python中的工具)。这里简单地介绍一种基于正则表达式的基本示例,用于提取HTML标签内的文本:
```cpp
#include <string>
#include <regex>
std::string extractContent(const std::string& html, const std::string& tag) {
std::regex pattern("(?i)<" + tag + ">(.*?)</" + tag + ">");
std::smatch match;
if (std::regex_search(html, match, pattern)) {
return match[1].str();
}
return "";
}
// 使用示例:
std::string html = "<div class='content'>Hello, World!</div>";
std::string extractedText = extractContent(html, "div");
```
这个函数接受一个HTML字符串和一个标签名(比如`div`),然后尝试查找匹配该标签的内容并返回。注意,这种方法仅适用于简单的HTML结构,并且对于复杂的嵌套和属性处理可能会有限。
相关问题
C++ 截取fileName字符串中最后一个“\”后的字符串
在C++中,你可以使用标准库中的`std::string`类来处理字符串,特别是`find_last_of`和`substr`这两个成员函数非常适合用来截取字符串中最后一个“\”后的子字符串。
具体步骤如下:
1. 使用`find_last_of`成员函数找到最后一个反斜杠“\”的索引位置。
2. 使用`substr`成员函数从找到的索引位置之后开始截取字符串,直到字符串的末尾。
下面是一个简单的代码示例:
```cpp
#include <iostream>
#include <string>
int main() {
std::string fileName = "C:\\path\\to\\file.txt";
std::string::size_type pos = fileName.find_last_of("\\");
if (pos != std::string::npos) {
std::string baseName = fileName.substr(pos + 1);
std::cout << "Base name is: " << baseName << std::endl;
} else {
std::cout << "No backslash found in string." << std::endl;
}
return 0;
}
```
这个程序会输出最后一个“\”之后的部分,即"file.txt"。
c++ 截取指定字符串后面的数据
在C++中,可以使用标准库中的字符串处理函数来截取指定字符串后面的数据。比如,可以使用`std::string::substr`函数来获取一个字符串的子串,然后使用`std::stoi`函数将子串转换为整数。以下是一个示例代码:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "hello 123 world";
std::string sub = str.substr(6);
int num = std::stoi(sub);
std::cout << "num = " << num << std::endl;
return 0;
}
```
在上面的示例代码中,`str`是原始字符串,`sub`是从第6个字符开始截取的子串,`std::stoi(sub)`将子串转换为整数并赋值给`num`,最后输出`num`的值为`123`。
阅读全文
相关推荐
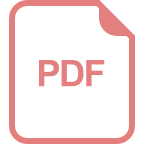
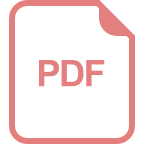
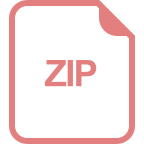











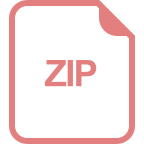
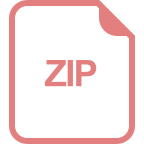