c++ string erase
时间: 2024-10-17 11:02:31 浏览: 38
在 C++ 中,`std::string` 类提供了一个 `erase` 方法,用于删除字符串中的指定部分或全部字符。这个方法有两个版本:
1. `erase(size_t pos)`:从位置 `pos` 开始删除直到末尾的所有字符。`pos` 参数是从 0 开始的索引。
2. `erase(size_t pos, size_t n)`:从位置 `pos` 开始并删除 `n` 个字符。这两个参数一起定义了要删除的字符范围。
例如,如果你想删除字符串中第一个 "world" 的所有字符,可以这样做:
```cpp
std::string str = "Hello, world!";
str.erase(str.find("world"), std::string::npos); // 使用find函数找到子串的位置,npos表示查找到末尾
```
如果你只是想删除特定位置的一个字符,只需要传递一个参数:
```cpp
str.erase(7); // 删除从第8个字符开始(因为索引从0开始)的单个字符
```
注意,`erase` 方法会改变原字符串,不会返回一个新的字符串。并且,如果试图从不存在的位置或超过字符串长度的地方删除字符,不会有任何效果。
相关问题
C++ string erase方法
在C++中,`string` 类的 `erase` 方法用于删除字符串中的某个子串或部分。该方法有几种不同的形式,可以按照需要选择。
`erase` 方法的基本语法如下:
```cpp
string& erase(size_type pos = 0, size_type count = npos);
```
* `pos`:要删除的子串的起始位置。
* `count`:要删除的字符数量。
下面是使用 `erase` 方法的一些例子:
1. 删除指定位置开始的特定数量的字符:
```cpp
std::string str = "Hello World!";
str.erase(7, 6); // 结果:"Hello "
```
在上述代码中,字符串 `"Hello World!"` 的从索引位置 7 开始(包括)到索引位置 7+6(不包括)的所有字符都被删除了。结果字符串为 "Hello "。
2. 删除子串:
```cpp
std::string str = "Hello World!";
str.erase("World"); // 结果:"Hello !"
```
在这个例子中,从 "Hello World!" 中删除了 "World",结果为 "Hello !"。注意,"World" 可以作为单个字符删除,如 `str.erase('W');`,结果是 "Hello !"。
以上是基本的 `erase` 方法用法。另外需要注意的是,在某些情况下,你可能会想要将其他数据类型转换为 `string` 类型后再使用 `erase` 方法,但是需要注意这种转换可能会导致问题(例如,可能会丢失一些信息)。如果你在不确定的情况下进行了这样的转换,你可能需要检查 `erase` 方法的行为是否符合你的预期。
c++ string.erase
The `string.erase()` function is a member function of the C++ standard library's `string` class. It is used to remove a portion of the string, starting at a specified position and for a specified length.
Syntax:
```
string.erase(position, length);
```
Here, `position` is an integer value that specifies the starting position from where the characters are to be removed. `length` is also an integer value that specifies the number of characters to be removed.
Example:
```c++
#include <iostream>
#include <string>
int main()
{
std::string str = "Hello, World!";
// remove the first 6 characters
str.erase(0, 6);
std::cout << str << std::endl; // output: World!
return 0;
}
```
In the above example, the `erase()` function is used to remove the first 6 characters from the string `str`. The resulting string is then output to the console.
阅读全文
相关推荐



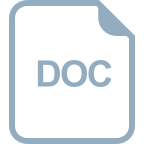











