board=[['.','6','1','.','3','.','.','2','.'], ['.','5','.','.','.','8','1','.','7'], ['.','.','.','.','.','7','.','3','4'], ['5','7','.','3','.','.','9','.','.'], ['1','9','.','7','.','.','.','.','.'], ['8','.','2','4','.','.','.','6','.'], ['.','4','.','.','1','.','2','5','.']] def check_node_choices(self, board, x, y): """ 检查节点所有可能性 """ choices = ['1', '2', '3', '4', '5', '6', '7', '8', '9'] for one in board[x]: if one in choices: choices.remove(one) for i in range(9): if board[i][y] in choices: choices.remove(board[i][y]) bx, by = (x // 3) * 3, (y // 3) * 3 for i in range(bx, bx + 3): for j in range(by, by+3): if board[i][j] in choices: choices.remove(board[i][j]) return choices for i in range(9): for j in range(9): if board[i][j] != '.': continue choices = self.check_node_choices(board, i, j) if not choices: pass while choices: board[i][j] = choices.pop() self.solveSudoku(board) if all('.' not in board[m] for m in range(i, 9)): pass board[i][j] = '.' if not choices: pass pass 这个程序怎么改正
时间: 2023-08-20 17:04:05 浏览: 43
根据您提供的代码,我注意到您正在尝试使用递归方法来解决数独问题。不过,我注意到您的代码中存在一些问题。首先,您定义了一个`check_node_choices`函数,但尝试在一个类中使用它作为实例方法。如果您想在类中使用它,应该将其定义为类方法或静态方法,并使用适当的修饰符。其次,您在循环中使用了`pass`语句,这会导致无限循环。最后,您没有提供完整的代码,因此我无法确定您的`solveSudoku`函数的实现。
以下是对您提供的代码进行修改和改正的示例:
```python
class SudokuSolver:
@staticmethod
def check_node_choices(board, x, y):
"""
检查节点所有可能性
"""
choices = ['1', '2', '3', '4', '5', '6', '7', '8', '9']
for one in board[x]:
if one in choices:
choices.remove(one)
for i in range(9):
if board[i][y] in choices:
choices.remove(board[i][y])
bx, by = (x // 3) * 3, (y // 3) * 3
for i in range(bx, bx + 3):
for j in range(by, by + 3):
if board[i][j] in choices:
choices.remove(board[i][j])
return choices
@staticmethod
def solve_sudoku(board):
for i in range(9):
for j in range(9):
if board[i][j] != '.':
continue
choices = SudokuSolver.check_node_choices(board, i, j)
if not choices:
return False
while choices:
board[i][j] = choices.pop()
if SudokuSolver.solve_sudoku(board):
return True
board[i][j] = '.'
return False
return True
# 创建一个 SudokuSolver 实例并调用 solve_sudoku 方法
solver = SudokuSolver()
solver.solve_sudoku(board)
```
通过以上修改,您应该能够正确地解决数独问题。请注意,我将`check_node_choices`函数和`solve_sudoku`函数定义为了静态方法,这意味着您无需实例化类就可以调用它们。另外,我还更正了循环中的`pass`语句,以避免无限循环的问题。
相关推荐
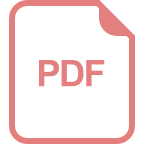
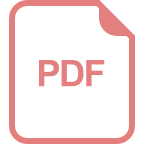
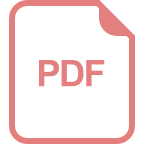
















