一段C语言程序,找出C:\folder\note\123.xlm文件中文本中的所有“”内的文字并打印
时间: 2024-12-19 07:20:19 浏览: 8
在C语言中,处理文件内容并搜索特定字符序列通常涉及到文件I/O操作和字符串处理函数。然而,由于C语言本身没有内置的功能直接读取Windows路径下的文件,我们通常会使用标准库`stdio.h`和`windows.h`配合使用。下面是一个简单的示例,假设你能打开和读取文件,这里我们将演示如何遍历文本查找双引号内的内容:
```c
#include <stdio.h>
#include <string.h>
#include <windows.h>
void find_quotes_in_file(const char* filename) {
HANDLE file = CreateFileA(filename, GENERIC_READ, FILE_SHARE_READ, NULL, OPEN_EXISTING, 0, NULL);
if (file == INVALID_HANDLE_VALUE) {
printf("Failed to open file %s\n", filename);
return;
}
DWORD fileSize;
FILETIME creationTime;
GetFileAttributesExA(filename, GetFileExInfoStandard, &creationTime);
if (!GetFileSize(file, &fileSize)) {
printf("Failed to get file size for %s\n", filename);
CloseHandle(file);
return;
}
char buffer[(int) fileSize + 1]; // Add extra space for null terminator
DWORD bytesRead;
if (!ReadFile(file, buffer, fileSize, &bytesRead, NULL)) {
printf("Failed to read file %s\n", filename);
CloseHandle(file);
return;
}
buffer[bytesRead] = '\0'; // Ensure the string is properly terminated
int start_index = 0;
bool inside_quotes = false;
for (size_t i = 0; i <= bytesRead - 1; i++) {
char c = buffer[i];
if (c == '\"') {
if (!inside_quotes) {
start_index = i + 1; // Start of quote block
inside_quotes = true;
} else {
inside_quotes = false;
printf("Found text in quotes: %.*s\n", (int)(i - start_index), buffer + start_index); // Print quoted text
}
}
}
CloseHandle(file);
}
// 使用函数:
int main() {
const char* filePath = "C:\\folder\\note\\123.txt"; // 假设是.txt文件
find_quotes_in_file(filePath);
return 0;
}
```
注意:这个例子假设文件是文本格式,并且双引号内的所有内容都是需要提取的。如果文件是二进制或者其他格式,上述代码可能无法正常工作。
阅读全文
相关推荐
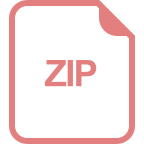
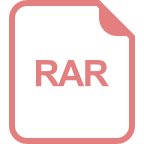
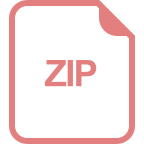

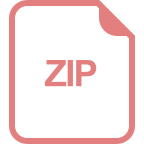
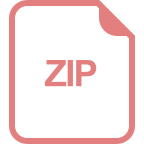
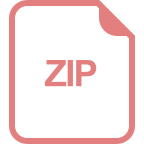
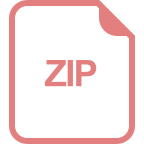
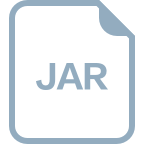
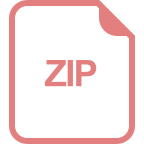
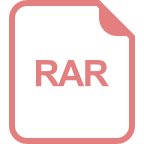
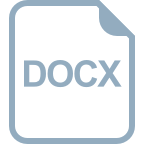
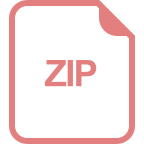
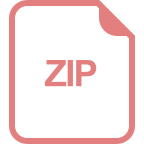
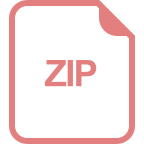
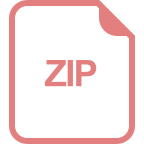
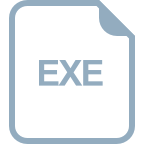
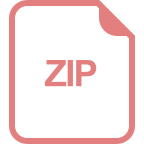