写一个三个同学的成绩找出最高成绩,按成绩高低排序的C语言
时间: 2024-10-03 18:03:14 浏览: 122
在C语言中,你可以使用结构体(struct)来表示学生的信息,并结合数组来存储多个学生的成绩。首先,定义一个包含姓名和成绩的结构体,然后创建一个结构体数组来存储三个同学的数据。接下来,遍历数组并找到最高分,最后按照成绩排序。以下是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
// 定义一个结构体表示学生及其成绩
typedef struct {
char name[50];
int score;
} Student;
// 比较函数用于分数排序
int compareStudents(const void *a, const void *b) {
return ((const Student *) a)->score - ((const Student *) b)->score;
}
int main() {
// 学生数组,假设有三个同学
Student students[3] = {
{"张三", 90},
{"李四", 98},
{"王五", 85}
};
// 找到最高分
int maxScore = students[0].score;
for (int i = 1; i < 3; i++) {
if (students[i].score > maxScore) {
maxScore = students[i].score;
}
}
printf("最高分为:%d 分,由 %s 获得\n", maxScore, students[1].name); // 注意索引,这里假设第二名最高
// 按照成绩从高到低排序
qsort(students, 3, sizeof(Student), compareStudents);
printf("成绩排序如下:\n");
for (int i = 0; i < 3; i++) {
printf("%s: %d 分\n", students[i].name, students[i].score);
}
return 0;
}
阅读全文
相关推荐
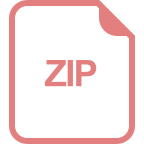
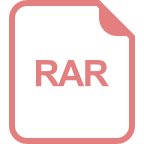
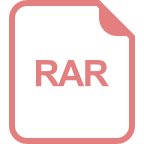
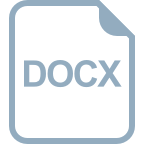
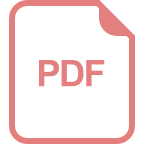
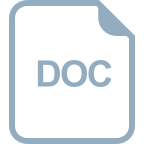
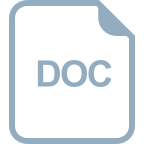
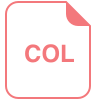
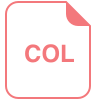
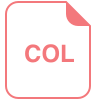

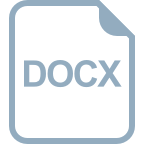
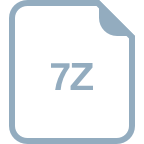
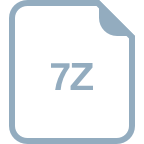
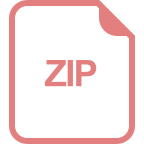