用c++写一个将文本文件内容读入单链表的模板函数
时间: 2024-10-13 15:03:05 浏览: 50
在C++中,我们可以使用`fstream`库来处理文件操作,并结合模板函数来创建一个通用的函数,该函数可以读取任意类型的字符数据并将其存储到单链表中。这里是一个简单的例子:
```cpp
#include <fstream>
#include <string>
#include <sstream>
template <typename T>
class Node {
public:
T data;
Node<T>* next;
Node(T val) : data(val), next(nullptr) {}
};
template <typename T>
void readTextFileToLinkedList(const std::string& filename, Node<T>** head) {
std::ifstream file(filename);
if (!file.is_open()) {
std::cerr << "Error opening the file." << std::endl;
return;
}
*head = nullptr; // 初始化链表头
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
T value;
iss >> value; // 将行转换成对应类型的数据
// 如果链表为空或当前节点不存在,新建一个节点
if (*head == nullptr) {
*head = new Node<T>(value);
} else {
Node<T>* current = *head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new Node<T>(value);
}
}
file.close();
}
// 使用示例:
int main() {
Node<char>** listHead;
readTextFileToLinkedList<std::string>("input.txt", &listHead);
// ...后续对链表的操作...
return 0;
}
```
这个模板函数`readTextFileToLinkedList`接受一个字符串类型的文件名以及一个指向`Node`指针的指针。函数会尝试打开文件、逐行读取,并将每一行的内容转换为指定类型(如`char`、`int`、`float`等),然后添加到单链表中。
阅读全文
相关推荐
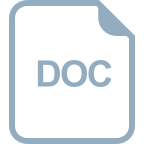
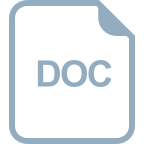
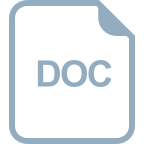
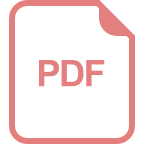
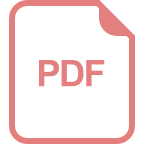
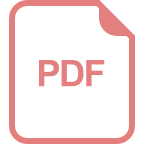
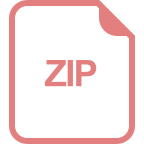
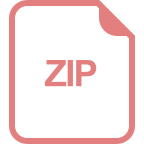
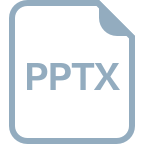
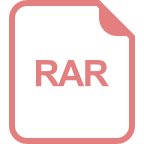
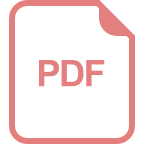
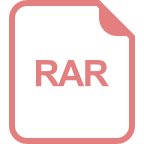
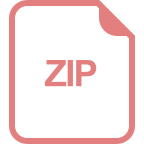
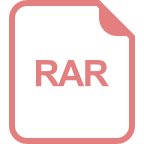