如何使用OpenCV在Python中创建RGB图像并实现鼠标选择兴趣区域后在新窗口展示该区域?
时间: 2024-11-04 12:11:32 浏览: 0
要使用OpenCV在Python中创建RGB图像并在新窗口展示鼠标选择的兴趣区域,你可以按照以下步骤操作:
1. 首先,确保已经安装了`opencv-python`库。如果没有,可以通过pip安装:
```
pip install opencv-python
```
2. 导入必要的OpenCV模块:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
```
3. 创建一个RGB图像,例如使用全黑背景:
```python
img = np.zeros((500, 500, 3), dtype=np.uint8) # (高度, 宽度, 帧数)
img[:, :] = (255, 0, 0) # 设置为红色(BGR色彩空间)
```
4. 显示原图,等待用户点击:
```python
cv2.imshow("Original Image", img)
cv2.waitKey(0) # 按任意键继续
```
5. 使用OpenCV的`waitKey`函数监听鼠标事件。当按下鼠标左键并移动时,记录下鼠标开始和结束的位置:
```python
start_pos = None
while True:
event, x, y, flags, _ = cv2.waitKeyEx(-1) & 0xFF
if event == cv2.EVENT_LBUTTONDOWN:
start_pos = (x, y)
elif event == cv2.EVENT_LBUTTONUP:
end_pos = (x, y)
break
if start_pos is not None and end_pos is not None:
# 计算选区范围
x1, y1 = start_pos
x2, y2 = end_pos
selection = img[y1:y2, x1:x2]
```
6. 创建一个新的窗口来显示选中的区域:
```python
new_window_name = "Selected Region"
cv2.namedWindow(new_window_name)
cv2.moveWindow(new_window_name, 0, 0) # 移动到屏幕顶部
cv2.imshow(new_window_name, selection)
cv2.waitKey(0) # 直到用户关闭窗口
```
7. 最后关闭所有窗口:
```python
cv2.destroyAllWindows()
```
完整代码如下:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 创建RGB图像
img = np.zeros((500, 500, 3), dtype=np.uint8)
img[:, :] = (255, 0, 0)
# 显示原图
cv2.imshow("Original Image", img)
cv2.waitKey(0)
start_pos = None
while True:
event, x, y, flags, _ = cv2.waitKeyEx(-1) & 0xFF
if event == cv2.EVENT_LBUTTONDOWN:
start_pos = (x, y)
elif event == cv2.EVENT_LBUTTONUP:
end_pos = (x, y)
break
if start_pos is not None and end_pos is not None:
x1, y1 = start_pos
x2, y2 = end_pos
selection = img[y1:y2, x1:x2]
# 新窗口显示选区
new_window_name = "Selected Region"
cv2.namedWindow(new_window_name)
cv2.moveWindow(new_window_name, 0, 0)
cv2.imshow(new_window_name, selection)
cv2.waitKey(0) # 用户关闭窗口前保持打开
cv2.destroyAllWindows()
```
现在,当你在原图上按住左键拖拽鼠标选择区域时,将在新窗口中看到该区域。
阅读全文
相关推荐
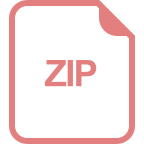
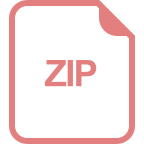
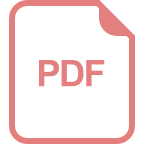
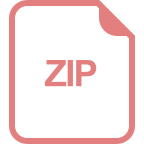
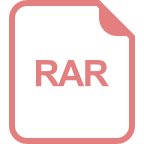
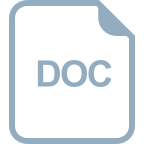
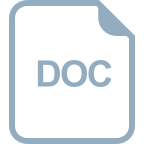
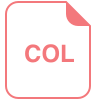
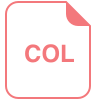
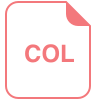
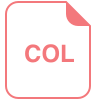
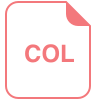
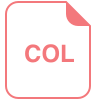
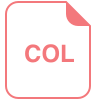
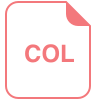