react封装hooks
时间: 2024-05-23 21:08:03 浏览: 228
React Hooks 是 React 16.8 的新增特性,它可以让我们在不编写类组件的情况下,使用 state 和其他 React 特性。而封装 Hooks 则是将一些常用的逻辑抽象出来,以自定义 Hooks 的形式提供给其他组件使用。封装 Hooks 可以提高代码的复用性和可维护性。
封装 Hooks 的步骤大致如下:
1. 确定封装的逻辑,将其抽象为一个自定义 Hook 函数。
2. 在 Hook 函数中使用 React Hooks API,如 useState、useEffect 等。
3. 将 Hook 函数暴露出去,供其他组件使用。
下面是一个简单的示例,封装了一个 useFetch 自定义 Hook,用于获取数据:
```
import { useState, useEffect } from 'react';
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
async function fetchData() {
try {
const response = await fetch(url);
const json = await response.json();
setData(json);
} catch (error) {
setError(error);
} finally {
setLoading(false);
}
}
fetchData();
}, [url]);
return { data, loading, error };
}
export default useFetch;
```
这个 useFetch Hook 封装了一个异步获取数据的逻辑。其他组件可以通过调用 useFetch 获取数据并进行渲染:
```
import React from 'react';
import useFetch from './useFetch';
function MyComponent() {
const { data, loading, error } = useFetch('https://jsonplaceholder.typicode.com/todos/1');
if (loading) {
return <div>Loading...</div>;
}
if (error) {
return <div>Error: {error.message}</div>;
}
return (
<div>
<h1>{data.title}</h1>
<p>{data.body}</p>
</div>
);
}
export default MyComponent;
```
阅读全文
相关推荐
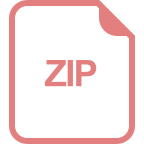
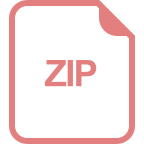
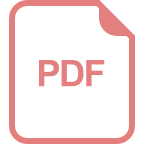

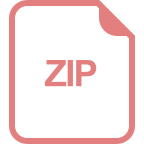
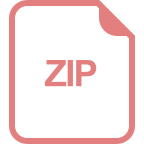

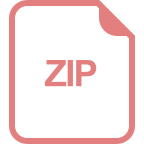
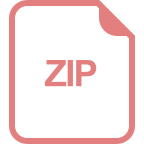
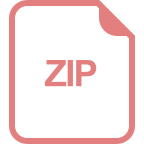
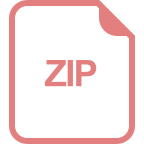
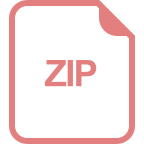
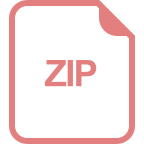
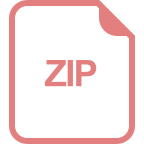
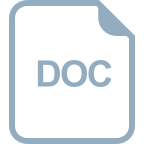
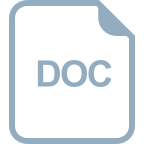


