用C++得到系统日历,判断当前年份是平年还是国年,判定当前月份是哪个季节
时间: 2024-09-18 17:15:53 浏览: 53
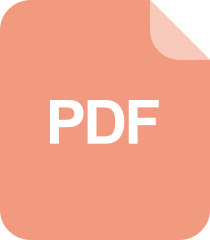
Java判断所给年份是平年还是闰年

在C++中,获取系统日历并检查年份是否为闰年并不直接内置在标准库中,但你可以利用`<ctime>`库来实现这一功能。首先,你需要包括头文件并创建一个`std::tm`结构来存储日期信息:
```cpp
#include <iostream>
#include <ctime>
bool is_leap_year(int year) {
if (year % 4 != 0)
return false;
else if (year % 100 != 0)
return true;
else if (year % 400 != 0)
return false;
else
return true;
}
int get_season(int month) {
static const int season_months[] = {0, 3, 6, 9}; // 春季、夏季、秋季、冬季的开始月份数
for (int i = 0; i < 4; ++i) {
if (month >= season_months[i])
return i + 1;
}
// 如果月份超过一年范围(12),返回春季,因为季节循环
return 1;
}
int main() {
time_t now = time(0);
struct tm *current_time = localtime(&now); // 获取当前时间
int current_year = current_time->tm_year + 1900; // 年份减去1900
int current_month = current_time->tm_mon + 1; // 月份加1(C++中的月份从0开始)
std::cout << "Current year: " << current_year << " is a leap year: " << (is_leap_year(current_year) ? "yes" : "no") << std::endl;
std::cout << "Current month: " << current_month << ", which corresponds to the " << get_season(current_month) << " season." << std::endl;
return 0;
}
```
上述代码首先定义了一个`is_leap_year`函数来检查给定年份是否为闰年,然后创建了`get_season`函数用于确定月份所属的季节。在`main`函数中,通过`time`和`localtime`函数获取当前系统时间,并计算相应的年份和月份。
阅读全文
相关推荐
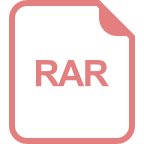















