set::intersection
时间: 2024-11-12 21:13:45 浏览: 23
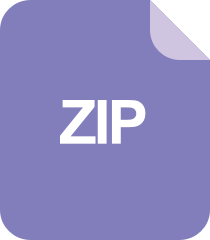
leetcode苹果-Set:放
在编程中,特别是使用集合数据结构如Python的set或C++ STL中的set时,`set::intersection`是一个成员函数,它的作用是获取两个集合(通常是set类型的)之间的交集。这个操作会返回一个新的集合,包含了原两个集合中共有的元素,即只包含那些在两个输入集合中都存在的元素。
例如,在Python中,你可以这样做:
```python
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
intersection = set1.intersection(set2)
print(intersection) # 输出:{3, 4}
```
同样,在C++中,如果你有一个`std::set<int>`的对象,可以这样操作:
```cpp
std::set<int> set1 = {1, 2, 3, 4};
std::set<int> set2 = {3, 4, 5, 6};
std::set<int> intersection = set1_intersection(set1, set2);
```
这里假设`set1_intersection`是实现了`std::set`间交集功能的一个自定义函数。
阅读全文
相关推荐
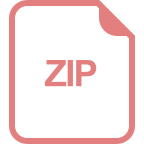
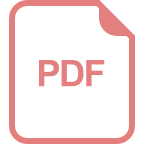
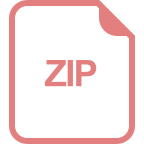
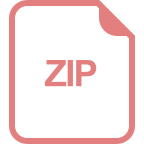
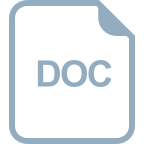









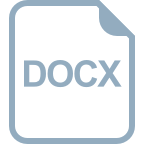
