输入一行字符,统计其中连续出现字符个数最多的情况,输出连续出现的那个字符,以及出现次数。 格式提醒:输入一行字符,输出连续出现字符个数最多的那个字符和出现次数,中间用空格隔开。
时间: 2024-10-23 15:15:30 浏览: 26
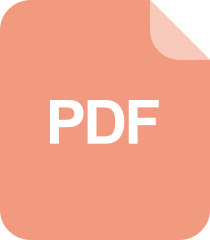
C++找出字符串中出现最多的字符和次数,时间复杂度小于O(n^2)
要解决这个问题,你可以使用一个简单的遍历算法,同时维护两个变量:一个记录当前最长连续字符(`max_char`)及其长度(`max_count`),另一个跟踪当前正在处理的字符(`current_char`)及其计数(`current_count`)。遍历过程中,如果遇到相同的字符,则增加计数;否则,比较新的计数和已知的最大计数,更新最大值。
下面是C++代码实现:
```cpp
#include <iostream>
#include <string>
std::pair<char, int> longest_consecutive_chars(const std::string& input) {
char max_char = input[0];
int max_count = 1;
char current_char = max_char;
int current_count = 1;
for (size_t i = 1; i < input.size(); ++i) {
if (input[i] == current_char) {
current_count++;
} else {
if (current_count > max_count) {
max_char = current_char;
max_count = current_count;
}
current_char = input[i];
current_count = 1;
}
}
// Check the last sequence
if (current_count > max_count) {
max_char = current_char;
max_count = current_count;
}
return std::make_pair(max_char, max_count);
}
int main() {
std::string line;
std::cout << "Enter a line of characters: ";
getline(std::cin, line);
std::pair<char, int> result = longest_consecutive_chars(line);
std::cout << "The character with the most consecutive appearances is '"
<< result.first << "', which appears " << result.second << " times.\n";
return 0;
}
```
当你运行这段程序时,它会提示你输入一行字符,然后输出连续出现次数最多的字符和它的出现次数。例如,如果你输入"aaabbbcccdd",输出将是'd'和4,因为'd'是最长连续出现的字符,出现了4次。
阅读全文
相关推荐
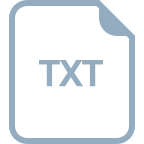
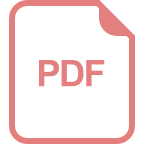
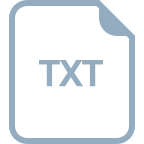
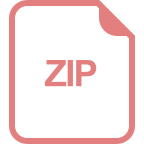
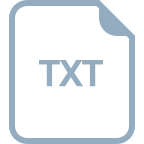
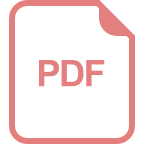
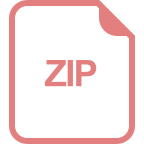
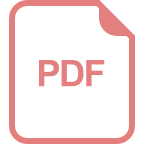
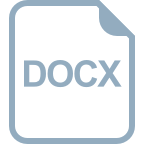
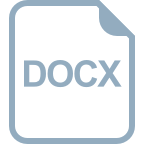
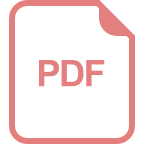
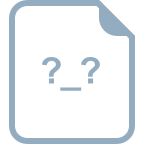
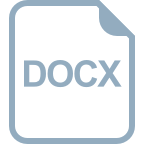
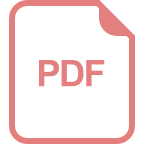