要求制作一个4人抢答器。无人抢答时,4个灯跑马循环,谁先按下,相对应的灯亮起,LED数码管显示抢答人编号,同时蜂鸣器发声。
时间: 2024-06-13 14:08:19 浏览: 155
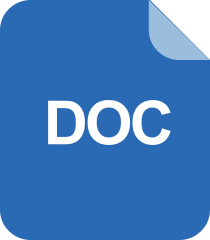
结合节能灯,彩灯循环,抢答器,声控闪光灯的电子技术课程设计
为了制作一个4人抢答器,需要以下步骤:
1.使用单片机作为核心,连接4个按钮S0-S3和一个系统清除和抢答控制开关S,以及4个LED灯和一个LED数码管和一个蜂鸣器。
2.设置一个系统清除和抢答控制开关S,开关由主持人控制。
3.使用锁存器来锁定抢答选手的编号,并在优先抢答选手的编号一直保持到主持人将系统清除为止。
4.使用定时器进行定时抢答功能,且一次抢答的时间由主持人设定(如20秒)。
5.当主持人启动“开始”键后,定时器进行减计时,同时扬声器发出短暂的声响,声响持续的时间为0.5s左右。
6.参赛选手在设定的时间内进行抢答,抢答有效,定时器停止工作,显示器上显示选手的编号和抢答的时间,并保持到主持人将系统清除为止。
7.如果在主持人启动"开始"键前抢答 ,抢答无效。且显示器上会显示抢答选手编号。
8.如果定时时间已到,无人抢答,本次抢答无效,系统报警并禁止抢答,定时显示器上显示00。
以下是一个简单的示例代码,仅供参考:
```c
#include <reg52.h>
#include <intrins.h>
#define uint unsigned int
#define uchar unsigned char
sbit S0 = P1^0;
sbit S1 = P1^1;
sbit S2 = P1^2;
sbit S3 = P1^3;
sbit LED0 = P2^0;
sbit LED1 = P2^1;
sbit LED2 = P2^2;
sbit LED3 = P2^3;
sbit BEEP = P2^4;
sbit LATCH = P2^5;
sbit CLK = P2^6;
sbit DIO = P2^7;
uchar code LED_DuanMa[17] = {0x3f, 0x06, 0x5b, 0x4f, 0x66, 0x6d, 0x7d, 0x07, 0x7f, 0x6f, 0x77, 0x7c, 0x39, 0x5e, 0x79, 0x71, 0x00};
uchar LED_Buffer[4] = {0xff, 0xff, 0xff, 0xff};
uchar LED_WeiMa[4] = {0xfe, 0xfd, 0xfb, 0xf7};
uchar Key_Buffer[4] = {0, 0, 0, 0};
uchar Key_Value[4] = {0x01, 0x02, 0x04, 0x08};
uchar Key_Flag = 0;
uchar Key_Num = 0;
uchar Time_Count = 0;
uchar Time_Flag = 0;
void Delay10ms(uint c) {
uchar a, b;
for (; c > 0; c--) {
for (b = 199; b > 0; b--) {
for (a = 1; a > 0; a--);
}
}
}
void Write_Latch(uchar dat) {
uchar i;
CLK = 0;
for (i = 0; i < 8; i++) {
DIO = dat & 0x80;
dat <<= 1;
CLK = 1;
_nop_();
_nop_();
CLK = 0;
}
LATCH = 1;
_nop_();
_nop_();
LATCH = 0;
}
void Display_LED() {
uchar i;
for (i = 0; i < 4; i++) {
Write_Latch(LED_Buffer[i]);
Write_Latch(LED_WeiMa[i]);
}
}
void Key_Scan() {
uchar i;
Key_Flag = 0;
if (S0 == 0 || S1 == 0 || S2 == 0 || S3 == 0) {
Delay10ms(1);
if (S0 == 0) {
Key_Buffer[0] |= Key_Value[0];
Key_Flag = 1;
}
if (S1 == 0) {
Key_Buffer[1] |= Key_Value[1];
Key_Flag = 1;
}
if (S2 == 0) {
Key_Buffer[2] |= Key_Value[2];
Key_Flag = 1;
}
if (S3 == 0) {
Key_Buffer[3] |= Key_Value[3];
Key_Flag = 1;
}
}
if (Key_Flag == 1) {
for (i = 0; i < 4; i++) {
if (Key_Buffer[i] != 0) {
Key_Num = i;
break;
}
}
}
}
void main() {
uchar i;
TMOD = 0x01;
TH0 = 0x3c;
TL0 = 0xb0;
TR0 = 1;
ET0 = 1;
EA = 1;
while (1) {
Key_Scan();
if (Key_Flag == 1) {
if (Time_Flag == 1) {
Time_Flag = 0;
Time_Count = 0;
for (i = 0; i < 4; i++) {
if (Key_Buffer[i] != 0) {
LED_Buffer[i] = LED_DuanMa[i + 1];
BEEP = 0;
Delay10ms(50);
BEEP = 1;
break;
}
}
}
for (i = 0; i < 4; i++) {
Key_Buffer[i] = 0;
}
}
Display_LED();
}
}
void Timer0() interrupt 1 {
TH0 = 0x3c;
TL0 = 0xb0;
Time_Count++;
if (Time_Count >= 200) {
Time_Flag = 1;
Time_Count = 0;
for (i = 0; i < 4; i++) {
LED_Buffer[i] = 0xff;
}
BEEP = 0;
Delay10ms(50);
BEEP = 1;
}
}
```
阅读全文
相关推荐
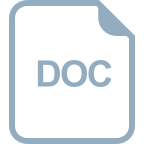
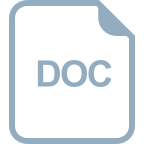



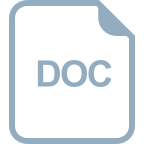
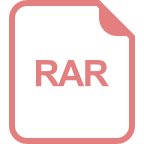
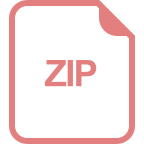
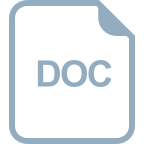
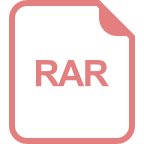
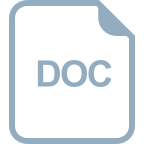
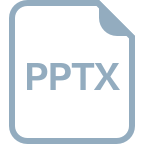
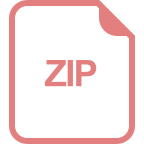
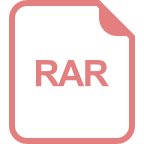
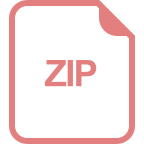
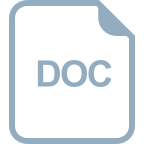
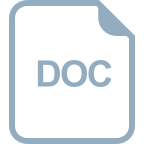
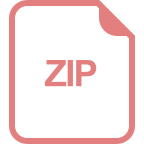