Kotlin操作sqlite
时间: 2024-12-10 21:47:12 浏览: 4
Kotlin是一门现代化的、静态类型的编程语言,它非常适合与SQLite这样的轻量级数据库一起工作。在Kotlin中操作SQLite通常涉及到以下步骤:
1. 添加依赖:首先,你需要在项目中引入SQLite的库。对于Android项目,可以使用KotlinX SQLite库,通过Gradle添加以下依赖:
```groovy
dependencies {
implementation "org.jetbrains.kotlinx:kotlinx-coroutines-android:1.x"
implementation "org.jetbrains.kotlinx:kotlinx-serialization-json:1.x"
implementation "org.jetbrains.exposed:exposed-core:0.x"
annotationProcessor "org.jetbrains.exposed:exposed-processor:0.x"
}
```
2. 创建数据模型(Entities):定义与SQLite表结构对应的Kotlin类,Exposed ORM是一个方便的选择,它可以自动生成SQL。
3. 连接到数据库:使用`Database.connect`函数建立连接到SQLite数据库,如:
```kotlin
val database = Database.connect("path/to/database.db")
```
4. 定义查询:使用Exposed的API编写CRUD(Create, Read, Update, Delete)操作,例如查询数据:
```kotlin
import org.jetbrains.exposed.sql.*
...
fun getUser(id: Int): User? {
return withTransaction {
select { User.id eq id }.singleOrNull()
}
}
// 更新数据
fun updateUser(user: User) {
update<User> { it.userId eq user.id } {
name = user.name,
email = user.email
}
}
```
5. 使用异步处理:由于SQLite操作是阻塞的,KotlinX Coroutines可以帮助你在主线程之外执行数据库操作,保持UI线程的响应式。
阅读全文
相关推荐
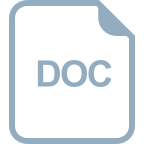
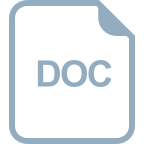
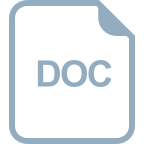
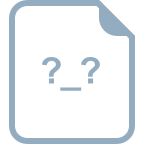
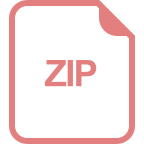
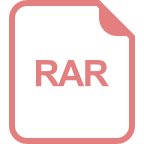
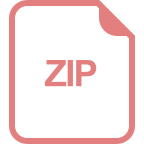
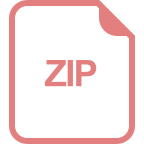
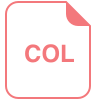



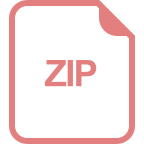


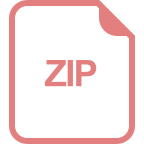
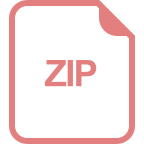

