编写函数删除字符串中的数字 c++
时间: 2024-12-20 10:23:20 浏览: 20
在C++中,你可以编写一个函数来删除字符串中的所有数字。这通常通过遍历字符串、检查字符是否为数字,并将其排除在外来完成。这里是一个简单的示例,它使用了`std::string`的`find_if`和`remove_if`功能:
```cpp
#include <algorithm>
#include <cctype>
std::string removeDigits(const std::string& str) {
std::string result;
std::copy_if(str.begin(), str.end(), std::back_inserter(result), ::isdigit);
return result;
}
// 示例用法
int main() {
std::string input = "abc123def456";
std::string output = removeDigits(input);
std::cout << "原始字符串: " << input << "\n";
std::cout << "处理后的字符串 (无数字): " << output << "\n";
return 0;
}
```
这个`removeDigits`函数会过滤掉字符串中的所有数字字符,只保留字母和其他非数字字符。
相关问题
编写一个函数fun利用函数删除字符串中的数字字符利用c++实现
好的,下面是一个利用函数删除字符串中数字字符的C++函数实现:
```c++
#include <iostream>
#include <string>
using namespace std;
bool isDigit(char c) {
return c >= '0' && c <= '9';
}
string fun(string s, bool (*isDigit)(char)) {
string res = "";
for (char c : s) {
if (!isDigit(c)) {
res += c;
}
}
return res;
}
int main() {
string s = "abc123def456";
string res = fun(s, isDigit);
cout << res << endl; // 输出"abcdef"
return 0;
}
```
该函数使用一个循环遍历输入字符串中的每个字符,如果该字符不是数字字符,则将其添加到结果字符串中。函数的第二个参数是一个指向函数的指针,该函数用于判断输入字符是否为数字字符。在主函数中,我们演示了如何使用该函数来删除字符串中的数字字符,并将结果输出到控制台。
编写一个函数fun其功能是删除字符串中的数字字符利用c++实现
好的,下面是一个删除字符串中数字字符的C++函数实现:
```c++
#include <iostream>
#include <string>
using namespace std;
string fun(string s) {
string res = "";
for (char c : s) {
if (!isdigit(c)) {
res += c;
}
}
return res;
}
int main() {
string s = "abc123def456";
string res = fun(s);
cout << res << endl; // 输出"abcdef"
return 0;
}
```
该函数使用一个循环遍历输入字符串中的每个字符,如果该字符不是数字字符,则将其添加到结果字符串中。最后,返回结果字符串作为函数的输出。在主函数中,我们演示了如何使用该函数来删除字符串中的数字字符,并将结果输出到控制台。
阅读全文
相关推荐








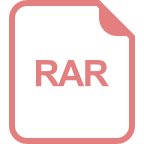







