BeanUtils.copyProperties复制boolean
时间: 2024-08-12 09:05:22 浏览: 26
`BeanUtils.copyProperties()` 是 Apache Commons BeanUtils 工具包提供的一个功能,用于将源对象(通常是 Map、Properties 或者已初始化的 Java bean)的属性值复制到目标对象上。对于 boolean 类型,`copyProperties()` 将直接进行赋值操作,即如果源对象的某个键对应的值是一个布尔值,它会把该布尔值设置为目标对象相应字段上。
例如,假设你有这样一个场景:
```java
Map<String, Object> source = new HashMap<>();
source.put("isEnabled", true); // 源 map 中有一个 boolean 值
MyTargetObject target = new MyTargetObject(); // 目标对象
BeanUtils.copyProperties(target, source);
```
在这个例子中,`isEnabled` 字段将会被 `target.isEnabled` 设定为 `true`。
需要注意的是,如果源值不是布尔类型,但在转换过程中能成功解析为布尔值(比如 "true"、"false"),则也能正常工作;但如果转换失败(如非字符串类型的无效值),可能会抛出异常。因此,在实际使用时,最好处理可能出现的异常。
相关问题
BeanUtils.copyProperties PropertyFilter
BeanUtils.copyProperties是Apache Commons BeanUtils库中的一个方法,用于将一个Java对象的属性值复制到另一个Java对象中。它可以方便地实现对象之间的属性拷贝,无需手动逐个设置属性值。
PropertyFilter是一个接口,用于在属性拷贝过程中进行过滤操作。它定义了一个方法,可以根据需要决定是否拷贝某个属性。通过实现PropertyFilter接口,可以自定义属性拷贝时的过滤规则。
以下是对BeanUtils.copyProperties和PropertyFilter的详细介绍:
1. BeanUtils.copyProperties:
- 作用:将源对象的属性值复制到目标对象中。
- 使用方法:BeanUtils.copyProperties(Object dest, Object orig)
- 参数说明:
- dest:目标对象,即要将属性值复制到的对象。
- orig:源对象,即要从中复制属性值的对象。
- 注意事项:
- 属性名和类型必须在源对象和目标对象中存在且匹配。
- 属性值会按照名称匹配进行复制,不会考虑类型转换。
2. PropertyFilter:
- 作用:在属性拷贝过程中进行过滤操作。
- 接口定义:org.apache.commons.beanutils.PropertyUtilsBean.PropertyFilter
- 方法定义:boolean apply(Object source, String name, Object value)
- 参数说明:
- source:源对象,即要从中复制属性值的对象。
- name:属性名。
- value:属性值。
- 返回值:
- true:拷贝该属性。
- false:不拷贝该属性。
BeanUtils.copyProperties忽略null值
可以使用BeanMapper或者在BeanUtils.copyProperties中传入一个自定义的属性过滤器来忽略null值。以下是两种方法的示例代码:
1. 使用BeanMapper忽略null值
```python
import net.sf.cglib.beans.BeanCopier;
import net.sf.cglib.core.Converter;
public class BeanMapper {
private static final Map<String, BeanCopier> BEAN_COPIERS = new ConcurrentHashMap<>();
public static void copyProperties(Object source, Object target) {
String key = source.getClass().toString() + target.getClass().toString();
BeanCopier copier = null;
if (!BEAN_COPIERS.containsKey(key)) {
copier = BeanCopier.create(source.getClass(), target.getClass(), true);
BEAN_COPIERS.put(key, copier);
} else {
copier = BEAN_COPIERS.get(key);
}
copier.copy(source, target, new Converter() {
@Override
public Object convert(Object value, Class target, Object context) {
if (value == null) {
if (target == String.class) {
return "";
} else if (target == Integer.class) {
return 0;
} else if (target == Long.class) {
return 0L;
} else if (target == Double.class) {
return 0.0;
} else if (target == Float.class) {
return 0.0f;
} else if (target == Boolean.class) {
return false;
} else {
return null;
}
} else {
return value;
}
}
});
}
}
```
使用示例:
```python
Source source = new Source();
source.setName("test");
source.setAge(18);
source.setAddress(null);
Target target = new Target();
BeanMapper.copyProperties(source, target);
System.out.println(target.getName()); // 输出:test
System.out.println(target.getAge()); // 输出:18
System.out.println(target.getAddress()); // 输出:null
```
2. 在BeanUtils.copyProperties中传入自定义属性过滤器忽略null值
```python
import org.springframework.beans.BeanUtils;
import org.springframework.beans.BeanWrapper;
import org.springframework.beans.BeanWrapperImpl;
import java.beans.PropertyDescriptor;
import java.util.HashSet;
import java.util.Set;
public class CopyUtils {
public static void copyPropertiesIgnoreNull(Object source, Object target) {
BeanUtils.copyProperties(source, target, getNullPropertyNames(source));
}
private static String[] getNullPropertyNames(Object source) {
final BeanWrapper src = new BeanWrapperImpl(source);
PropertyDescriptor[] pds = src.getPropertyDescriptors();
Set<String> emptyNames = new HashSet<>();
for (PropertyDescriptor pd : pds) {
Object srcValue = src.getPropertyValue(pd.getName());
if (srcValue == null) {
emptyNames.add(pd.getName());
}
}
String[] result = new String[emptyNames.size()];
return emptyNames.toArray(result);
}
}
```
使用示例:
```python
Source source = new Source();
source.setName("test");
source.setAge(18);
source.setAddress(null);
Target target = new Target();
CopyUtils.copyPropertiesIgnoreNull(source, target);
System.out.println(target.getName()); // 输出:test
System.out.println(target.getAge()); // 输出:18
System.out.println(target.getAddress()); // 输出:null
```
相关推荐
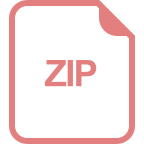
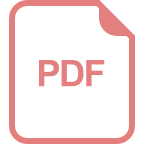
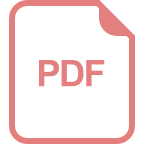













