1.添加考题(无限制添加)题目,4个答案,正确答案,每题分数 2.生成试卷(随机抽取考题,形成试卷)100分,考题不能重复 用Java写
时间: 2024-04-14 21:29:12 浏览: 97
你好!对于你的需求,我可以给出一个简单的Java代码示例来实现添加考题和生成试卷的功能。以下是代码示例:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
// 考题类
class Question {
private String title; // 题目
private List<String> options; // 选项
private int correctAnswer; // 正确答案(选项的索引)
private int score; // 分数
public Question(String title, List<String> options, int correctAnswer, int score) {
this.title = title;
this.options = options;
this.correctAnswer = correctAnswer;
this.score = score;
}
public String getTitle() {
return title;
}
public List<String> getOptions() {
return options;
}
public int getCorrectAnswer() {
return correctAnswer;
}
public int getScore() {
return score;
}
}
// 试卷生成器类
class ExamPaperGenerator {
private List<Question> allQuestions; // 所有考题
public ExamPaperGenerator() {
allQuestions = new ArrayList<>();
}
// 添加考题
public void addQuestion(Question question) {
allQuestions.add(question);
}
// 生成试卷
public List<Question> generateExamPaper() {
List<Question> examPaper = new ArrayList<>();
Random random = new Random();
while (examPaper.size() < 10) { // 假设试卷中有10道题
Question randomQuestion = allQuestions.get(random.nextInt(allQuestions.size()));
if (!examPaper.contains(randomQuestion)) {
examPaper.add(randomQuestion);
}
}
return examPaper;
}
}
public class Main {
public static void main(String[] args) {
ExamPaperGenerator generator = new ExamPaperGenerator();
// 添加考题
Question question1 = new Question("问题1", List.of("选项A", "选项B", "选项C", "选项D"), 2, 10);
Question question2 = new Question("问题2", List.of("选项A", "选项B", "选项C", "选项D"), 0, 5);
// 添加更多的考题...
generator.addQuestion(question1);
generator.addQuestion(question2);
// 生成试卷
List<Question> examPaper = generator.generateExamPaper();
// 打印试卷
for (int i = 0; i < examPaper.size(); i++) {
Question question = examPaper.get(i);
System.out.println("第" + (i + 1) + "题:" + question.getTitle());
System.out.println("选项:" + question.getOptions());
// 其他打印信息,如:正确答案、分数等
}
}
}
```
这段代码演示了如何使用Java实现添加考题和生成试卷的功能。你可以根据自己的需求进行修改和扩展。希望对你有所帮助!如果有任何问题,请随时向我提问。
阅读全文
相关推荐
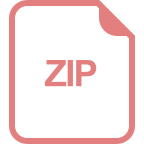
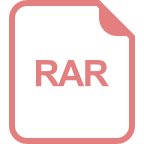
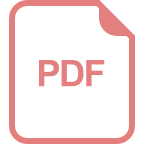
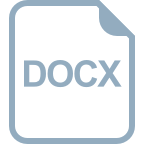

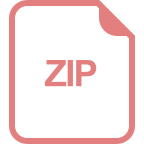
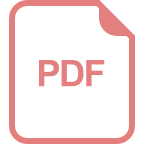