用c语言产生50个1到100的随机整数,并将其中的偶数保存到一个数组中
时间: 2024-09-11 15:06:06 浏览: 19
在C语言中,你可以使用`<stdlib.h>`库的`srand()`函数设置随机数种子,然后用`rand()`函数生成随机数,结合`%`运算符保证只取范围内的整数。对于找出并保存偶数,可以创建一个数组专门存储偶数。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h> // 使用时间作为随机数种子
#define MAX_NUM 100
#define ARRAY_SIZE 25 // 假设我们想要存储50个偶数,因为1到100内有50个偶数
int main() {
int random_array[ARRAY_SIZE]; // 存储偶数的数组
int count = 0; // 记录已找到的偶数数量
srand(time(0)); // 设置随机数种子,增加随机性
for (int i = 0; i < 50 && count < ARRAY_SIZE; i++) {
int num = rand() % MAX_NUM + 1; // 生成1到MAX_NUM之间的随机数
if (num % 2 == 0) { // 检查是否是偶数
random_array[count] = num;
count++;
}
}
// 打印结果
printf("偶数数组:\n");
for (int i = 0; i < count; i++) {
printf("%d ", random_array[i]);
}
return 0;
}
```
在这个程序中,我们循环50次,每次生成一个随机数,如果它是偶数就添加到数组中。注意由于数组大小限制,如果产生的随机数过多超过数组容量,这个程序会提前结束。
相关推荐
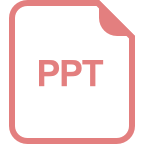
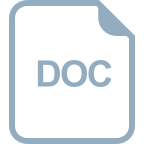
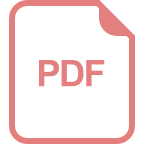
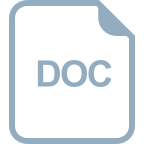
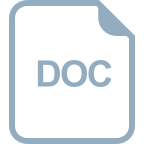












