java实现抢票程序
时间: 2024-06-16 08:04:57 浏览: 390
Java可以通过多线程来实现抢票程序。以下是一个简单的Java抢票程序的实现示例:
```java
import java.util.concurrent.atomic.AtomicInteger;
public class TicketSystem {
private static AtomicInteger tickets = new AtomicInteger(10);
public static void main(String[] args) {
// 创建多个线程模拟多个用户抢票
for (int i = 1; i <= 5; i++) {
Thread thread = new Thread(new User(i));
thread.start();
}
}
static class User implements Runnable {
private int userId;
public User(int userId) {
this.userId = userId;
}
@Override
public void run() {
while (true) {
int remainingTickets = tickets.get();
if (remainingTickets > 0) {
// 模拟用户抢票的过程
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
// 使用CAS操作进行票数减一
if (tickets.compareAndSet(remainingTickets, remainingTickets - 1)) {
System.out.println("用户" + userId + "抢到了一张票,剩余票数:" + (remainingTickets - 1));
break;
}
} else {
System.out.println("用户" + userId + "没有抢到票");
break;
}
}
}
}
}
```
上述代码中,我们使用了`AtomicInteger`来保证票数的原子性操作,避免了多个线程同时修改票数导致的问题。每个用户通过一个线程来模拟抢票的过程,当剩余票数大于0时,用户线程会尝试使用CAS操作将票数减一,如果成功则表示抢到了一张票,否则继续循环直到抢票成功或者票已售罄。
阅读全文
相关推荐
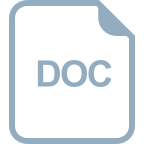
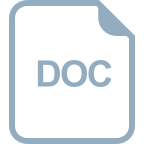
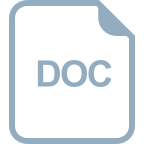
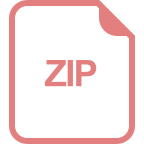
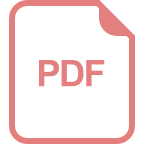
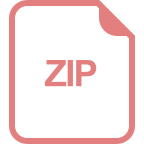
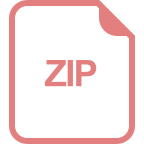
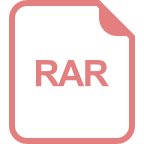
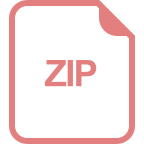
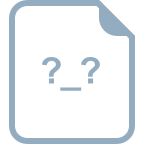
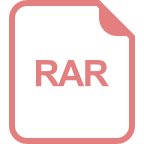
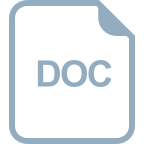






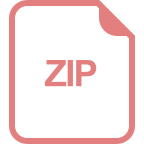