vs创建一个单链表L,依次插入11、12、13、14、15、16、17、18八个元素,输出单链表L各结点的数据域。
时间: 2024-09-28 20:07:04 浏览: 30
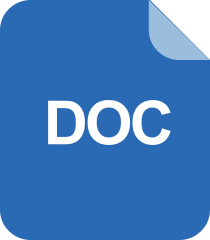
单链表的应用,多项式的合并

在C++中,你可以使用结构体(struct)来表示链表节点,并创建一个动态单链表。首先定义一个链表节点结构,例如:
```cpp
struct ListNode {
int data;
ListNode* next; // 指向下一个节点的指针
ListNode(int val = 0) : data(val), next(nullptr) {} // 构造函数设置初始值
};
```
然后你可以按照以下步骤创建并操作这个单链表:
1. 创建头节点(假设初始为空):
```cpp
ListNode* head = nullptr;
```
2. 分别插入数据:
```cpp
head = insert(head, 11); // 插入第一个元素
head = insert(head, 12);
head = insert(head, 13);
// ... 继续添加剩余元素
head = insert(head, 18);
```
3. 定义插入函数:
```cpp
ListNode* insert(ListNode*& head, int value) {
ListNode* newNode = new ListNode(value);
if (head == nullptr) {
head = newNode;
} else {
ListNode* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
return head;
}
```
4. 输出链表节点数据:
由于链表是单向的,你需要遍历整个链表才能打印每个节点的数据。这里提供一个简单的遍历和打印函数示例:
```cpp
void printList(ListNode* node) {
while (node != nullptr) {
cout << node->data << " ";
node = node->next;
}
cout << endl;
}
```
最后,调用`printList(head)`即可打印出链表的所有节点数据。
阅读全文
相关推荐
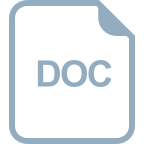
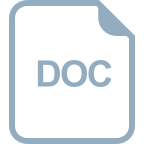











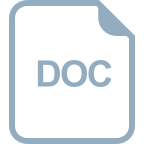
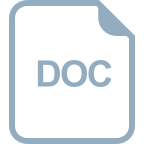
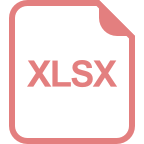
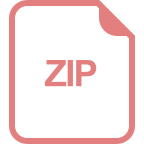
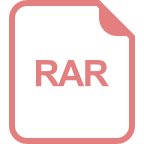
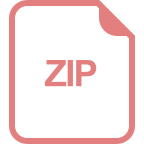