std::function与std::bind结合使用体现出多态性的例子
时间: 2023-07-23 11:05:38 浏览: 101
当`std::function`与`std::bind`结合使用时,可以实现多态性,即在运行时动态地选择不同的函数或成员函数进行调用。下面是一个使用示例:
```cpp
#include <iostream>
#include <functional>
void printMessage(const std::string& message) {
std::cout << "Message: " << message << std::endl;
}
class Person {
public:
void greet(const std::string& name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
};
int main() {
std::function<void()> func1 = std::bind(printMessage, "Hello, World!");
func1(); // 输出结果为: Message: Hello, World!
Person person;
std::function<void(const std::string&)> func2 = std::bind(&Person::greet, &person, std::placeholders::_1);
func2("Alice"); // 输出结果为: Hello, Alice!
return 0;
}
```
在上面的示例中,我们首先定义了一个名为`printMessage`的函数,它接受一个`std::string`类型的参数并打印消息。然后,我们定义了一个名为`Person`的类,它有一个成员函数`greet`,接受一个`std::string`类型的参数并打印问候语。
在`main`函数中,我们首先使用`std::bind`将函数`printMessage`绑定到`func1`上,并传递参数"Hello, World!"。然后,我们通过调用`func1`来执行绑定的函数,输出结果为"Message: Hello, World!"。
接下来,我们创建了一个`Person`对象`person`,然后使用`std::bind`将成员函数`greet`绑定到`func2`上。我们使用`std::placeholders::_1`占位符来表示在调用时传递的参数。最后,我们通过调用`func2`来执行绑定的成员函数,并传递参数"Alice",输出结果为"Hello, Alice!"。
通过使用`std::function`和`std::bind`,我们可以将不同类型的函数和成员函数绑定到相同类型的对象上,从而实现多态性,并在运行时动态选择不同的函数进行调用。
阅读全文
相关推荐
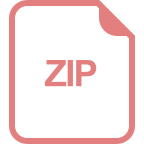
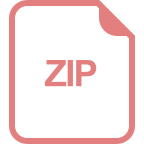
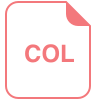
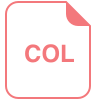
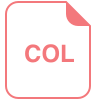
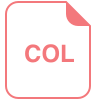
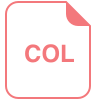
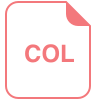
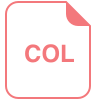
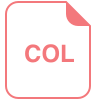
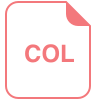
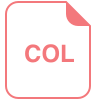
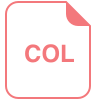
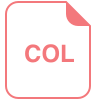
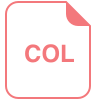
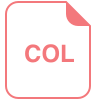
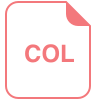
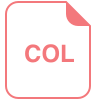