银行排队模型代码c++
时间: 2023-12-10 21:32:35 浏览: 99
银行排队模型是一种常见的事件驱动模型,它可以用来模拟银行等服务场所的排队情况。以下是一个简单的C++代码实现:
```
#include <iostream>
#include <queue>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAX_WINDOW_NUM = 10; // 最大窗口数
const int MAX_CUSTOMER_NUM = 100; // 最大顾客数
// 顾客类
class Customer {
public:
Customer(int arrive_time = 0, int service_time = 0) :
arrive_time_(arrive_time), service_time_(service_time) {}
int arrive_time() const { return arrive_time_; }
int service_time() const { return service_time_; }
private:
int arrive_time_; // 到达时间
int service_time_; // 服务时间
};
// 窗口类
class Window {
public:
Window() : is_busy_(false), current_customer_(NULL) {}
bool is_busy() const { return is_busy_; }
void serveCustomer(Customer& customer) {
is_busy_ = true;
current_customer_ = &customer;
}
void finishService() {
is_busy_ = false;
current_customer_ = NULL;
}
Customer* current_customer() const { return current_customer_; }
private:
bool is_busy_; // 是否忙碌
Customer* current_customer_; // 当前服务的顾客
};
// 队列系统类
class QueueSystem {
public:
QueueSystem(int total_service_time, int window_num) :
total_service_time_(total_service_time), window_num_(window_num),
current_time_(0), customer_num_(0), total_customer_wait_time_(0) {
srand(time(NULL));
for (int i = 0; i < window_num_; ++i) {
windows_[i] = Window();
}
}
void simulate() {
while (current_time_ < total_service_time_) {
generateCustomer();
updateWindow();
++current_time_;
}
printStatistics();
}
private:
void generateCustomer() {
if (rand() % 3 == 0) { // 每秒钟有1/3的概率生成一个新顾客
Customer customer(current_time_, rand() % 5 + 1);
customer_queue_.push(customer);
++customer_num_;
}
}
void updateWindow() {
for (int i = 0; i < window_num_; ++i) {
if (windows_[i].is_busy()) { // 如果窗口正在服务顾客
Customer* customer = windows_[i].current_customer();
if (current_time_ - customer->arrive_time() >= customer->service_time()) { // 如果顾客服务结束
windows_[i].finishService();
total_customer_wait_time_ += current_time_ - customer->arrive_time() - customer->service_time();
}
}
if (!windows_[i].is_busy() && !customer_queue_.empty()) { // 如果窗口空闲且队列中有顾客
Customer customer = customer_queue_.front();
customer_queue_.pop();
windows_[i].serveCustomer(customer);
}
}
}
void printStatistics() {
cout << "顾客总数:" << customer_num_ << endl;
cout << "平均等待时间:" << (double)total_customer_wait_time_ / customer_num_ << endl;
}
private:
int total_service_time_; // 总服务时间
int window_num_; // 窗口数
int current_time_; // 当前时间
int customer_num_; // 顾客总数
int total_customer_wait_time_; // 顾客总等待时间
Window windows_[MAX_WINDOW_NUM]; // 窗口数组
queue<Customer> customer_queue_; // 顾客队列
};
int main() {
QueueSystem queue_system(60, 3); // 总服务时间为60秒,3个窗口
queue_system.simulate();
return 0;
}
```
阅读全文
相关推荐
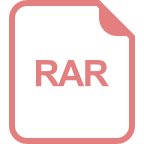
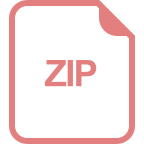
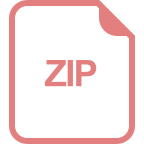
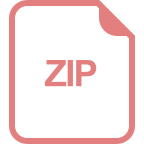
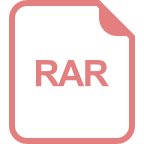
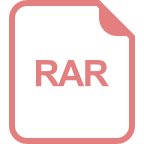
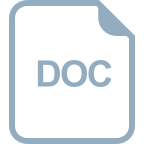
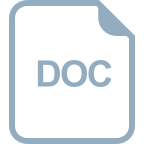
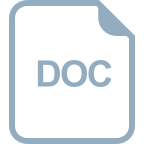
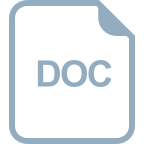
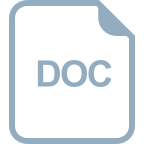
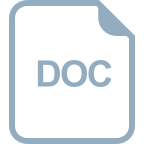
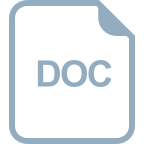
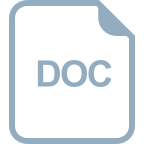
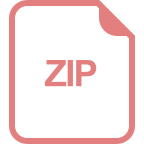