kernel_read与 vfs_read
时间: 2024-01-13 22:03:38 浏览: 312
kernel_read()和vfs_read()都是Linux内核中用于读取文件的函数,但它们的使用场景略有不同。
vfs_read()是内核中用于读取文件的标准函数,可以在内核中的任何地方使用。它的函数原型如下:
```c
ssize_t vfs_read(struct file *file, char __user *buf, size_t count, loff_t *pos);
```
其中,file是要读取的文件,buf是读取数据的缓冲区,count是要读取的字节数,pos是文件中的偏移量。vfs_read()函数会从文件的当前位置开始读取count个字节的数据到buf中,并返回实际读取的字节数。
而kernel_read()函数则是在文件系统中实现的一个特殊函数,只能在文件系统的read()函数中使用。它的函数原型如下:
```c
ssize_t kernel_read(struct file *file, void *buf, size_t count, loff_t *pos);
```
其中,file是要读取的文件,buf是读取数据的缓冲区,count是要读取的字节数,pos是文件中的偏移量。kernel_read()函数会从文件的当前位置开始读取count个字节的数据到buf中,并返回实际读取的字节数。
因此,如果你需要在文件系统的read()函数中读取文件,应该使用kernel_read()函数;如果你需要在内核的其他地方读取文件,应该使用vfs_read()函数。
相关问题
安卓内核使用vfs_read如何提取data/brint的文件内容并传递给int类型的数据
在 Android 内核中,可以使用 vfs_read 函数从文件系统中读取文件内容。具体步骤如下:
1. 首先打开 data/brint 文件,可以使用类似下面的代码:
```
struct file *filp;
char *filename = "/data/brint";
filp = filp_open(filename, O_RDONLY, 0);
if (IS_ERR(filp)) {
printk(KERN_ALERT "Failed to open file %s\n", filename);
return -ENOENT;
}
```
2. 然后创建一个缓冲区 buf,将文件内容读取到缓冲区中:
```
char buf[1024];
unsigned int len = 0;
mm_segment_t old_fs = get_fs();
set_fs(get_ds());
len = vfs_read(filp, buf, sizeof(buf), &filp->f_pos);
set_fs(old_fs);
```
3. 最后将读取到的字符串转换成 int 类型的数据:
```
int num = 0;
kstrtoint(buf, 10, &num);
```
完整代码如下:
```
#include <linux/fs.h>
#include <linux/kernel.h>
#include <linux/module.h>
#include <linux/uaccess.h>
#define BRINT_FILE "/data/brint"
static int __init my_init(void)
{
struct file *filp;
char buf[1024];
unsigned int len = 0;
int num = 0;
mm_segment_t old_fs;
// Open the file
filp = filp_open(BRINT_FILE, O_RDONLY, 0);
if (IS_ERR(filp)) {
printk(KERN_ALERT "Failed to open file %s\n", BRINT_FILE);
return -ENOENT;
}
// Read the file content
old_fs = get_fs();
set_fs(get_ds());
len = vfs_read(filp, buf, sizeof(buf), &filp->f_pos);
set_fs(old_fs);
// Convert the string to int
kstrtoint(buf, 10, &num);
printk(KERN_INFO "Read %d from file %s\n", num, BRINT_FILE);
filp_close(filp, NULL);
return 0;
}
static void __exit my_exit(void)
{
}
module_init(my_init);
module_exit(my_exit);
MODULE_LICENSE("GPL");
```
kernel读写文件
在 Linux 内核中,可以使用以下函数来读写文件:
1. `filp_open()` 函数:用于打开文件,返回一个指向打开文件对象的指针。
2. `vfs_read()` 函数:用于从文件中读取数据。
3. `vfs_write()` 函数:用于向文件中写入数据。
4. `filp_close()` 函数:用于关闭文件。
以下是一个简单的示例,展示如何在内核中读取和写入文件:
```c
#include <linux/fs.h>
#include <asm/uaccess.h>
static int read_file(char *filename, char *buffer, int buflen)
{
struct file *f;
loff_t pos = 0;
ssize_t ret;
f = filp_open(filename, O_RDONLY, 0);
if (IS_ERR(f))
return -1;
ret = vfs_read(f, buffer, buflen, &pos);
filp_close(f, NULL);
return ret;
}
static int write_file(char *filename, char *buffer, int buflen)
{
struct file *f;
loff_t pos = 0;
ssize_t ret;
f = filp_open(filename, O_WRONLY | O_CREAT, 0644);
if (IS_ERR(f))
return -1;
ret = vfs_write(f, buffer, buflen, &pos);
filp_close(f, NULL);
return ret;
}
```
在上面的示例中,`read_file()` 函数使用 `filp_open()` 函数打开指定的文件,然后使用 `vfs_read()` 函数从文件中读取数据。`write_file()` 函数使用 `filp_open()` 函数打开指定的文件,然后使用 `vfs_write()` 函数向文件中写入数据。最后,使用 `filp_close()` 函数关闭文件。
需要注意的是,由于内核空间和用户空间的地址空间是隔离的,因此在内核中读取和写入文件时,需要使用 `copy_from_user()` 和 `copy_to_user()` 函数来进行数据的复制。
阅读全文
相关推荐
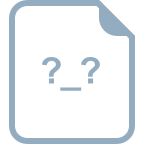
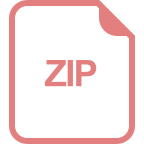
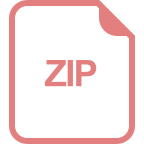
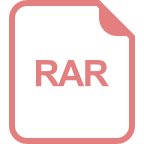
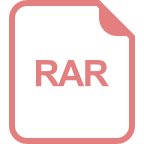
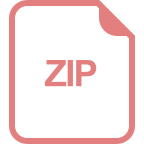
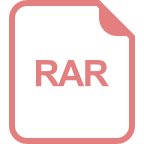
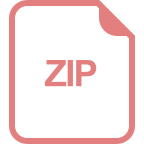
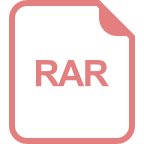
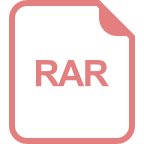
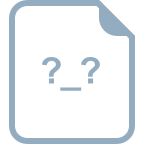
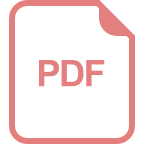
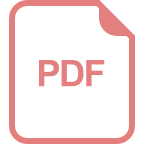
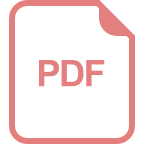
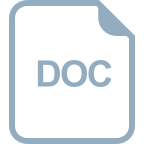
