db.stdinfo.find({"stu_sex":"女","course_score.java":{$gt:85},},{"stu_name":1,"course_score.java":1,"stu_sex":1});
时间: 2023-11-26 11:49:03 浏览: 32
这是一个MongoDB的查询语句,用于在名为stdinfo的数据库中查找满足条件的学生信息。具体来说,它会返回所有性别为女且Java课程成绩大于85分的学生的姓名、Java课程成绩和性别信息。其中,db表示当前数据库,stdinfo表示集合名称,find()方法用于查找符合条件的文档,第一个参数是查询条件,第二个参数是指定要返回的字段,1表示要返回该字段,0表示不返回该字段。具体语法如下:
```
db.stdinfo.find(
{"stu_sex": "女", "course_score.java": {"$gt": 85}},
{"stu_name": 1, "course_score.java": 1, "stu_sex": 1}
)
```
相关问题
db.stdinfo.update({"stu_face":"null"}, {$set:{"stu_face":"群众"}});
这是一个MongoDB数据库的更新操作,它的作用是将名为stdinfo的集合中所有stu_face字段值为"null"的文档的stu_face字段值更新为"群众"。具体解释如下:
- db.stdinfo.update:表示对名为stdinfo的集合进行更新操作。
- {"stu_face":"null"}:表示筛选出所有stu_face字段值为"null"的文档。
- {$set:{"stu_face":"群众"}}:表示将筛选出的文档的stu_face字段值更新为"群众"。
如果你想在JSP中使用MySQL数据库,可以参考以下代码:
```java
<%@ page import="java.sql.*" %>
<%
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 加载数据库驱动
Class.forName("com.mysql.jdbc.Driver");
// 获取数据库连接
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
// 创建Statement对象
stmt = conn.createStatement();
// 执行SQL语句
rs = stmt.executeQuery("SELECT * FROM student");
// 处理查询结果
while (rs.next()) {
out.println(rs.getString("name"));
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stmt != null) {
try {
stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
%>
```
问题描述】结构体应用。全班有30位同学,某学期的课程数量为4门,利用结构体存储学生的基本信息(包括学号、姓名、4门成绩、绩点),通过从键盘上输入30个同学的学号、姓名、4门成绩,计算出每个同学的绩点,输出所有同学的信息(学号、姓名、4门成绩、绩点),并输出绩点最高的同学的学号和姓名。其中,存储学生信息的结构体为: struct stdinfo { char no[20]; char name[10]; float score[4]; float gpa; //绩点 }; 这里假设每个人的绩点为各科绩点的总和,单科成绩可通过下述公式转换为对应绩点值。 若单科成绩小于60,则单科绩点等于0;若单科成绩大于或等于60,则单科绩点等于1+(单科成绩-60)/10.例如,成绩50分对应绩点0,成绩62对应绩点1.2,成绩85对应3.5 【输入形式】 【输出形式】标题名Id、Name、Score1、Score2、Score3、Score4、GPA用\t分隔; 一行中的数据也用\t分隔; 【输入/输出样例】红色部分代表输入的信息。 Please enter students number:5 Please input all student information: 101 s01 88 68 76 80 102 s02 78 80 88 69 103 s03 50 68 62 55 104 s04 90 99 92 95 105 s05 62 71 80 75 Id Name Score1 Score2 Score3 Score4 gpa 101 s01 88.00 68.00 76.00 80.00 11.20 102 s02 78.00 80.00 88.00 69.00 11.50 103 s03 50.00 68.00 62.00 55.00 3.00 104 s04 90.00 99.00 92.00 95.00 17.60 105 s05 62.00 71.00 80.00 75.00 8.80 The highest gpa is 17.60, Id is 104, name is s04.
在 C 语言中,可以使用如下代码来实现结构体的存储和计算:
```
#include <stdio.h>
#include <stdlib.h>
#define STUDENT_NUM 30 // 学生数量
#define COURSE_NUM 4 // 课程数量
// 定义结构体
struct stdinfo {
char no[20]; // 学号
char name[10]; // 姓名
float score[4]; // 成绩
float gpa; // 绩点
};
int main()
{
int i, j;
float highest_gpa = 0.0; // 最高绩点
int highest_index = 0; // 最高绩点的学生编号
struct stdinfo students[STUDENT_NUM]; // 学生信息
// 输入学生信息
printf("Please enter students number:%d\n", STUDENT_NUM);
printf("Please input all student information:\n");
for (i = 0; i < STUDENT_NUM; i++) {
scanf("%s%s", students[i].no, students[i].name); // 输入学号和姓名
for (j = 0; j < COURSE_NUM; j++) {
scanf("%f", &students[i].score[j]); // 输入成绩
}
}
// 计算绩点
for (i = 0; i < STUDENT_NUM; i++) {
float gpa = 0.0; // 绩点
for (j = 0; j < COURSE_NUM; j++) {
if (students[i].score[j] < 60) { // 小于60分
gpa += 0.0;
} else { // 大于等于60分
gpa += (students[i].score[j] - 60) / 10.0;
}
}
students[i].gpa = gpa; // 记录绩点
// 更新最高绩点信息
if (gpa > highest_gpa) {
highest_gpa = gpa;
highest_index = i;
}
}
// 输出所有学生信息
printf("Id\tName\tScore1\tScore2\tScore3\tScore4\tgpa\n");
for (i = 0; i < STUDENT_NUM; i++) {
printf("%s\t%s\t%.2f\t%.2f\t
相关推荐
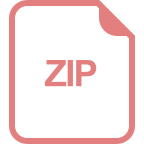
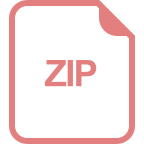
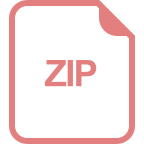
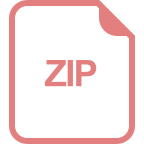
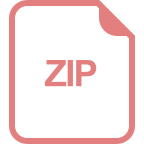
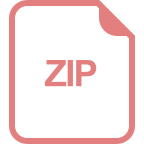
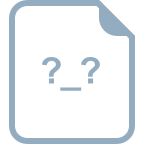
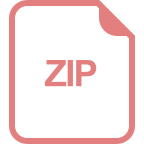