写一段c语言代码,实现多次设置矩形区域并叠加
时间: 2024-12-20 12:25:40 浏览: 4
在C语言中,我们可以使用结构体和函数来表示矩形区域,并通过动态内存分配来实现多次添加和叠加矩形的功能。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义矩形区域结构体
typedef struct {
int x1; // 左上角x坐标
int y1; // 左上角y坐标
int width; // 宽度
int height; // 高度
} Rectangle;
// 函数用于绘制矩形
void draw_rectangle(Rectangle *rect) {
printf("Rectangle at (%d,%d) with width %d and height %d\n", rect->x1, rect->y1, rect->width, rect->height);
}
// 功能函数,增加并叠加新的矩形
void add_and_overlap(Rectangle **rects, int count, Rectangle new_rect) {
// 检查是否需要扩容
if (count == 0 || new_rect.x1 <= rects[count - 1]->x1 + rects[count - 1]->width) {
// 如果需要,动态分配存储空间
if (count > 0)
realloc(rects, sizeof(Rectangle*) * (count + 1));
rects[count] = &new_rect;
count++;
} else {
// 如果新矩形与现有矩形不相邻,则单独绘制
draw_rectangle(&new_rect);
}
}
int main() {
Rectangle *rects = NULL;
int num_rects = 0;
// 添加第一个矩形
add_and_overlap(&rects, num_rects, (Rectangle){10, 10, 50, 30});
// 添加第二个矩形,它会覆盖第一个矩形的一部分
add_and_overlap(&rects, num_rects, (Rectangle){40, 20, 60, 40});
// 打印所有矩形
for (int i = 0; i < num_rects; i++) {
draw_rectangle(rects + i);
}
return 0;
}
```
阅读全文
相关推荐





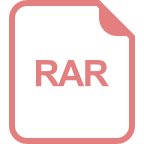





