C# 如何设置winfrom 全屏显示
时间: 2024-09-28 14:14:07 浏览: 56
在C#中,为了使Windows Forms应用程序全屏显示,你可以使用Form类的ShowWithoutDialog属性以及FormBorderStyle属性。以下是一个简单的示例:
```csharp
using System.Windows.Forms;
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
this.FormBorderStyle = FormBorderStyle.None; // 设置无边框
this.ShowWithoutDialog(); // 将窗体显示而非对话框形式,实现全屏
}
}
```
在这个例子中,我们首先设置`FormBorderStyle`为`None`,去除默认的边框。然后使用`ShowWithoutDialog()`代替传统的`Show()`方法,使得窗体以全屏模式显示。
注意,这种方式会让窗体覆盖整个桌面,而不是像任务栏通知那样仅占据一部分屏幕。用户可以通过按下Alt+Enter组合键退出全屏模式。
相关问题
c#在winfrom里面怎么设置按键录制视频全屏
你可以使用C#中的Screen类来截取屏幕,然后使用AVIFile库将截取的屏幕帧保存为AVI格式的视频文件。以下是一个简单的示例代码:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using System.Runtime.InteropServices;
namespace ScreenRecorder
{
public partial class Form1 : Form
{
[DllImport("user32.dll")]
static extern IntPtr GetDesktopWindow();
[DllImport("user32.dll")]
static extern IntPtr GetWindowDC(IntPtr hWnd);
[DllImport("user32.dll")]
static extern IntPtr ReleaseDC(IntPtr hWnd, IntPtr hDC);
[DllImport("avifil32.dll")]
static extern void AVIFileInit();
[DllImport("avifil32.dll")]
static extern void AVIFileExit();
[DllImport("avifil32.dll")]
static extern int AVIFileOpenW(ref IntPtr ppfile, [MarshalAs(UnmanagedType.LPWStr)]string szFile, int uMode, int pclsidHandler);
[DllImport("avifil32.dll")]
static extern int AVIFileCreateStream(IntPtr pfile, out IntPtr ppavi, ref AVISTREAMINFOW psi);
[DllImport("avifil32.dll")]
static extern int AVIStreamSetFormat(IntPtr avi, int lPos, ref BITMAPINFOHEADER lpFormat, int cbFormat);
[DllImport("avifil32.dll")]
static extern int AVIStreamWrite(IntPtr pavi, int lStart, int lSamples, IntPtr lpBuffer, int cbBuffer, int dwFlags, int dummy1, int dummy2);
[DllImport("avifil32.dll")]
static extern int AVIStreamRelease(IntPtr pavi);
[DllImport("avifil32.dll")]
static extern int AVIFileRelease(IntPtr pfile);
[StructLayout(LayoutKind.Sequential, Pack = 1)]
struct BITMAPINFOHEADER
{
public int biSize;
public int biWidth;
public int biHeight;
public short biPlanes;
public short biBitCount;
public int biCompression;
public int biSizeImage;
public int biXPelsPerMeter;
public int biYPelsPerMeter;
public int biClrUsed;
public int biClrImportant;
}
[StructLayout(LayoutKind.Sequential, Pack = 1)]
struct AVISTREAMINFOW
{
public int fccType;
public int fccHandler;
public int dwFlags;
public int dwCaps;
public short wPriority;
public short wLanguage;
public int dwScale;
public int dwRate;
public int dwStart;
public int dwLength;
public int dwInitialFrames;
public int dwSuggestedBufferSize;
public int dwQuality;
public int dwSampleSize;
public short left;
public short top;
public short right;
public short bottom;
}
IntPtr aviFile = IntPtr.Zero;
IntPtr aviStream = IntPtr.Zero;
BITMAPINFOHEADER bih = new BITMAPINFOHEADER();
public Form1()
{
InitializeComponent();
}
private void btnStart_Click(object sender, EventArgs e)
{
AVIFileInit();
// 打开AVI文件
AVIFileOpenW(ref aviFile, "test.avi", 4097, 0);
// 设置流格式
bih.biSize = Marshal.SizeOf(typeof(BITMAPINFOHEADER));
bih.biWidth = Screen.PrimaryScreen.Bounds.Width;
bih.biHeight = Screen.PrimaryScreen.Bounds.Height;
bih.biPlanes = 1;
bih.biBitCount = 24;
bih.biCompression = 0;
bih.biSizeImage = bih.biWidth * bih.biHeight * 3;
AVISTREAMINFOW strhdr = new AVISTREAMINFOW();
strhdr.fccType = 1935960438;
strhdr.fccHandler = 0;
strhdr.dwFlags = 0;
strhdr.dwCaps = 0;
strhdr.wPriority = 0;
strhdr.wLanguage = 0;
strhdr.dwScale = 1;
strhdr.dwRate = 25;
strhdr.dwStart = 0;
strhdr.dwLength = 0;
strhdr.dwInitialFrames = 0;
strhdr.dwSuggestedBufferSize = bih.biSizeImage;
strhdr.dwQuality = -1;
strhdr.dwSampleSize = 0;
strhdr.left = 0;
strhdr.top = 0;
strhdr.right = (short)bih.biWidth;
strhdr.bottom = (short)bih.biHeight;
AVIFileCreateStream(aviFile, out aviStream, ref strhdr);
AVIStreamSetFormat(aviStream, 0, ref bih, bih.biSize);
timer1.Start();
}
private void btnStop_Click(object sender, EventArgs e)
{
timer1.Stop();
AVIStreamRelease(aviStream);
AVIFileRelease(aviFile);
AVIFileExit();
}
private void timer1_Tick(object sender, EventArgs e)
{
IntPtr hdcSrc = GetWindowDC(GetDesktopWindow());
IntPtr hdcDest = bih.biHeight < 0 ? CreateCompatibleDC(hdcSrc) : CreateCompatibleDC(IntPtr.Zero);
IntPtr hBitmap = CreateCompatibleBitmap(hdcSrc, Screen.PrimaryScreen.Bounds.Width, Screen.PrimaryScreen.Bounds.Height);
IntPtr hOld = SelectObject(hdcDest, hBitmap);
BitBlt(hdcDest, 0, 0, Screen.PrimaryScreen.Bounds.Width, Screen.PrimaryScreen.Bounds.Height, hdcSrc, 0, 0, 13369376);
AVIStreamWrite(aviStream, timer1.Interval * timer1.TickCount, 1, hBitmap, bih.biSizeImage, 0, 0, 0);
SelectObject(hdcDest, hOld);
DeleteObject(hBitmap);
DeleteDC(hdcDest);
ReleaseDC(IntPtr.Zero, hdcSrc);
}
}
}
```
在上面的代码中,我们使用了Windows API函数来截取屏幕,然后使用AVIFile库将帧写入AVI文件。你可以修改代码来控制录制的区域、帧率和保存的文件名等参数。
C# WinForm 技巧十: winfrom 全屏自适应屏幕分辨率
要实现 WinForm 全屏自适应屏幕分辨率,可以使用以下代码:
```csharp
this.WindowState = FormWindowState.Maximized;
this.FormBorderStyle = FormBorderStyle.None;
this.Bounds = Screen.PrimaryScreen.Bounds;
```
其中,`FormWindowState.Maximized` 用于将窗口最大化,`FormBorderStyle.None` 用于取消窗口边框,`Screen.PrimaryScreen.Bounds` 用于获取主屏幕的边界信息,然后将窗口大小设置为屏幕大小。
另外,如果想要在窗口大小变化时自动调整控件位置和大小,可以在 `Form.Resize` 事件中编写相关代码。例如:
```csharp
private void Form1_Resize(object sender, EventArgs e)
{
// 根据窗口大小调整控件位置和大小
button1.Left = (this.ClientSize.Width - button1.Width) / 2;
button1.Top = (this.ClientSize.Height - button1.Height) / 2;
}
```
在这个例子中,`button1` 是一个按钮控件,当窗口大小变化时,通过计算居中的方式调整按钮的位置。
阅读全文
相关推荐

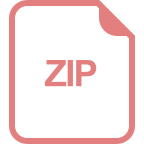
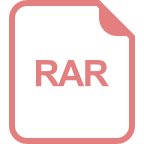
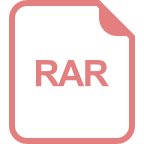
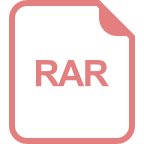
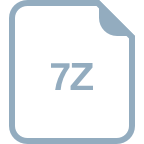
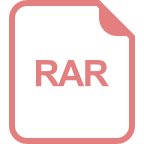
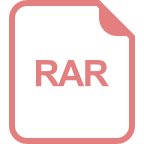
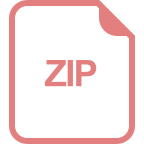
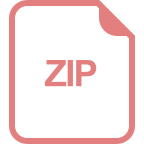
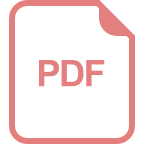
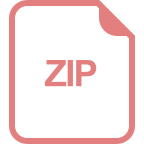
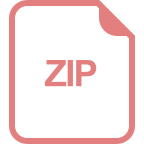
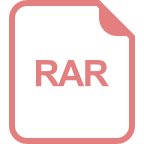