vue3 父组件传值到子组件
时间: 2024-09-19 18:01:31 浏览: 36
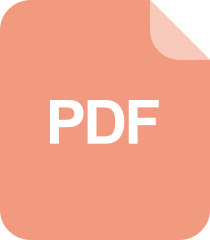
vue2.0 子组件改变props值,并向父组件传值的方法
Vue3 中,父组件向子组件传递数据通常有以下几种方式:
1. **props**(属性):这是最常见的父子组件通信方式。父组件通过`props`将数据作为参数传递给子组件。子组件在 `setup()` 钩子中声明需要接收的 props,并可以在模板上直接使用它们。
```vue
// 父组件
<template>
<child-component :value="parentValue" />
</template>
<script setup>
import ChildComponent from './ChildComponent.vue';
const parentValue = 'Hello from parent';
</script>
// 子组件
<template>
<div>{{ value }}</div>
</template>
<script setup>
import { ref } from 'vue';
export default {
props: ['value'],
setup(props) {
const localValue = ref(props.value); // 在子组件中可以修改值,但不会影响父组件
return {};
}
};
```
2. **自定义事件**($emit/$on):当子组件需要通知父组件更新某个状态时,可以发射自定义事件,父组件通过 `$on` 监听这个事件。
```vue
// 子组件
<button @click="$emit('update:parentValue', newValue)">Update Value</button>
// 父组件
<template>
<child-component v-on:update:parentValue="handleParentValueChange"></child-component>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
methods: {
handleParentValueChange(newValue) {
this.parentValue = newValue;
},
},
};
```
3. **ref 和 reactive**:如果需要频繁地双向绑定,Vue 提供了 `reactive` 和 `ref` 来创建响应式的数据结构,然后通过它们互相传递。
```vue
// 父组件
<script setup>
import { ref } from 'vue';
const parentValue = ref('Hello');
</script>
// 子组件
<template>
<input type="text" v-model:bind="$parent.parentValue" />
</template>
```
阅读全文
相关推荐
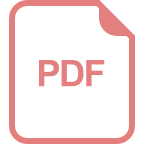
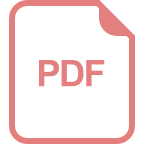














