bmp图像边缘检测c语言
时间: 2023-11-07 07:47:50 浏览: 143
边缘检测是图像处理中常用的技术之一,可以帮助我们找到图像中的边界区域。在C语言中,我们可以使用一些基本的算法来实现边缘检测,如Sobel算子和Canny算子。
以下是一个使用Sobel算子进行边缘检测的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 512
#define HEIGHT 512
void sobelEdgeDetection(unsigned char* image, unsigned char* result) {
int Gx[3][3] = {{-1, 0, 1}, {-2, 0, 2}, {-1, 0, 1}};
int Gy[3][3] = {{-1, -2, -1}, {0, 0, 0}, {1, 2, 1}};
for (int y = 1; y < HEIGHT - 1; y++) {
for (int x = 1; x < WIDTH - 1; x++) {
int sumX = 0;
int sumY = 0;
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
int pixel = image[(y + i) * WIDTH + (x + j)]; sumX += pixel * Gx[i + 1][j + 1];
sumY += pixel * Gy[i + 1][j + 1];
}
}
int magnitude = abs(sumX) + abs(sumY);
result[y * WIDTH + x] = (magnitude > 128) ? 255 : 0; // 使用阈值来二值化结果
}
}
}
int main() {
FILE* file = fopen("input.bmp", "rb");
unsigned char* image = (unsigned char*)malloc(WIDTH * HEIGHT);
fread(image, 1, WIDTH * HEIGHT, file);
fclose(file);
unsigned char* result = (unsigned char*)malloc(WIDTH * HEIGHT);
sobelEdgeDetection(image, result);
FILE* outfile = fopen("output.bmp", "wb");
fwrite(result, 1, WIDTH * HEIGHT, outfile);
fclose(outfile);
free(image);
free(result);
return 0;
}
```
以上代码使用Sobel算子进行边缘检测,并将结果保存为一幅二值化的图像。你可以将需要处理的图像命名为"input.bmp",运行程序后将会生成名为"output.bmp"的结果图像。请注意,这里的图像格式是灰度图像,每个像素点用一个字节表示灰度值(0-255)。
阅读全文
相关推荐

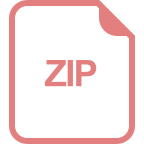

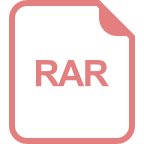




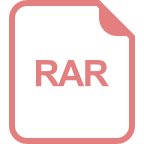
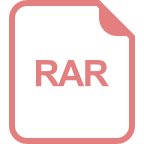




