写一段C++代码,实现读写格式化文本配置文件
时间: 2024-04-10 21:33:51 浏览: 31
当然,我可以帮你写一段C++代码来实现读写格式化文本配置文件。以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <map>
// 函数用于读取配置文件
std::map<std::string, std::string> readConfigFile(const std::string& filename) {
std::map<std::string, std::string> config;
std::ifstream file(filename);
std::string line;
while (std::getline(file, line)) {
// 忽略空行和注释
if (line.empty() || line[0] == '#') {
continue;
}
std::istringstream iss(line);
std::string key, value;
if (std::getline(iss, key, '=') && std::getline(iss, value)) {
config[key] = value;
}
}
return config;
}
// 函数用于写入配置文件
void writeConfigFile(const std::string& filename, const std::map<std::string, std::string>& config) {
std::ofstream file(filename);
for (const auto& pair : config) {
file << pair.first << "=" << pair.second << std::endl;
}
}
int main() {
// 读取配置文件
std::map<std::string, std::string> config = readConfigFile("config.txt");
// 输出读取到的配置
for (const auto& pair : config) {
std::cout << pair.first << " = " << pair.second << std::endl;
}
// 修改配置
config["key1"] = "new_value1";
config["key2"] = "new_value2";
// 写入配置文件
writeConfigFile("config.txt", config);
return 0;
}
```
上述代码实现了读取和写入格式化文本配置文件的功能。你可以根据自己的需求修改文件名和配置项。读取配置文件的函数`readConfigFile`使用了`std::map`来存储键值对,写入配置文件的函数`writeConfigFile`则根据`std::map`的内容将配置项写入文件中。在示例代码中,我们假设配置文件名为`config.txt`,你可以根据实际情况修改文件名。
希望这段代码能够帮助到你!如果有任何疑问,请随时提问。
相关推荐
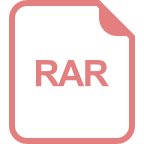
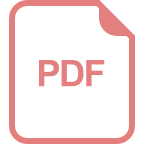














