Android 读取Excel
时间: 2023-10-13 19:44:57 浏览: 47
在Android中读取Excel文件可以使用Apache POI库。下面是一个简单的示例代码,演示如何读取Excel文件中的数据:
首先,需要在项目的`build.gradle`文件中添加POI库的依赖:
```groovy
dependencies {
implementation 'org.apache.poi:poi:4.1.2'
implementation 'org.apache.poi:poi-ooxml:4.1.2'
}
```
然后,可以使用以下代码读取Excel文件:
```java
import org.apache.poi.ss.usermodel.*;
import java.io.FileInputStream;
import java.io.IOException;
public class ExcelReader {
public static void main(String[] args) {
try {
FileInputStream file = new FileInputStream("path/to/your/excel/file.xlsx");
Workbook workbook = WorkbookFactory.create(file);
Sheet sheet = workbook.getSheetAt(0); // 获取第一个工作表
for (Row row : sheet) {
for (Cell cell : row) {
CellType cellType = cell.getCellType();
switch (cellType) {
case STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case BOOLEAN:
System.out.print(cell.getBooleanCellValue() + "\t");
break;
default:
System.out.print("\t");
break;
}
}
System.out.println();
}
file.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
替换代码中的`"path/to/your/excel/file.xlsx"`为你实际的Excel文件路径。以上代码将打印出Excel文件中每个单元格的值。
请注意,这只是一个简单的示例,实际应用中可能需要处理更复杂的Excel文件结构和数据类型。
相关推荐
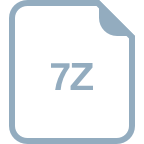
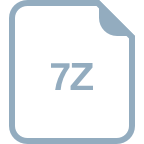














