c# 如何通过WINFORM程序向控制台程序输出参数 并将控制台程序的输出结果输入到winform
时间: 2024-10-06 22:01:13 浏览: 9
在 C# 中,你可以使用 `Process` 类来创建、管理和操作外部应用程序,包括 WinForm 向控制台程序传递参数以及接收控制台程序的输出。以下是基本步骤:
1. **创建控制台程序**:首先,编写一个控制台应用程序,它接受命令行参数并返回一些输出。例如,你可以有一个简单的控制台程序 `ConsoleApp.exe`:
```csharp
// 控制台App.cs
using System;
namespace ConsoleApp
{
class Program
{
static void Main(string[] args)
{
if (args.Length > 0)
Console.WriteLine("Received argument: " + args[0]);
else
Console.WriteLine("No argument provided.");
}
}
}
```
2. **WinForm 程序传递参数**:在 WinForm 中,通过 `Process.Start` 方法启动控制台程序,并传递参数:
```csharp
using System.Diagnostics;
using System.Windows.Forms;
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
// 在按钮点击事件中调用
button1.Click += Button1_Click;
}
private void Button1_Click(object sender, EventArgs e)
{
ProcessStartInfo psi = new ProcessStartInfo("ConsoleApp.exe", "YourArgument");
psi.UseShellExecute = false; // 如果在Windows上,设置为true会打开一个新的进程窗口
using (Process process = new Process())
{
process.StartInfo = psi;
process.Start();
// 通常在这里读取控制台输出,但这通常是异步的,所以你需要等待或使用OutputDataReceived事件
// process.StandardOutput.ReadToEnd(); 这一行需要处理异步情况,这里省略
}
}
}
```
3. **接收控制台输出**:控制台程序的输出一般不会立即返回给 WinForm,因为它们是在独立线程中运行的。为了获取输出,你可以使用 `StandardOutput` 属性,并订阅 `OutputDataReceived` 事件:
```csharp
private void process_OutputDataReceived(object sender, DataReceivedEventArgs e)
{
if (!string.IsNullOrEmpty(e.Data))
{
textBoxOutput.AppendText(e.Data);
}
}
// ... 在启动过程后添加这行代码
process.BeginOutputReadLine();
```
相关推荐
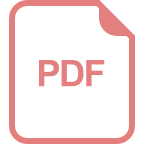
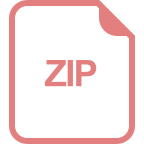
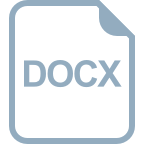














