Qt6中编写dll动态库,并使用基类和派生类,在参数不同时调用不同的dll文件
时间: 2024-12-15 17:16:37 浏览: 3
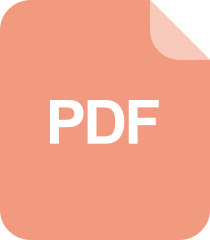
C++基类指针和派生类指针之间的转换方法讲解
在Qt 6中,如果你需要编写DLL (动态链接库) 并利用基类和派生类提供多种功能,可以按照以下步骤操作:
1. **创建基类 DLL**:
首先,在基类中,设计好公共接口,包括纯虚函数,这将作为所有派生类的基础。例如:
```cpp
// BaseClass.h
class BaseClass {
public:
virtual void process(int type) = 0; // 纯虚函数,将在派生类中实现
};
```
2. **派生类及其DLL实现**:
每个派生类都负责实现`process`函数,并且针对不同的参数类型做处理。例如,`DerivedClass1.dll` 和 `DerivedClass2.dll`:
```cpp
// DerivedClass1.cpp
extern "C" __declspec(dllexport) void process(int type) override { ... } // 类型1的处理
```
```cpp
// DerivedClass2.cpp
extern "C" __declspec(dllexport) void process(int type) override { ... } // 类型2的处理
```
3. **主程序引用和动态加载**:
在主应用程序中,你可以使用`QLibrary`或者直接通过`LoadLibrary`函数加载对应的DLL,然后通过指针来调用派生类的方法。比如:
```cpp
#include <QCoreApplication>
#include <QLibrary>
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
// 加载指定类型的DLL
QLibrary lib("path_to_DerivedClass1.dll");
if (!lib.load()) {
qCritical() << "Failed to load library";
return -1;
}
// 获取BaseClass的指针并调用过程
BaseClass* derivedClass = static_cast<BaseClass*>(lib.resolve("process"));
if (!derivedClass) {
qCritical() << "Failed to resolve function pointer";
return -1;
}
derivedClass->process(1); // 调用DerivedClass1的版本
// 同理,可以按需加载其他DLL
return app.exec();
}
阅读全文
相关推荐
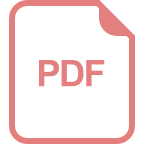
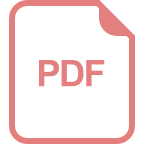
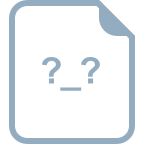
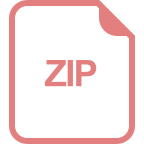
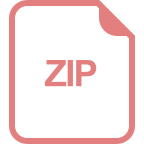
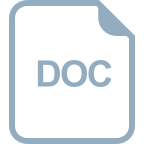
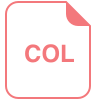
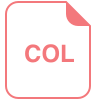
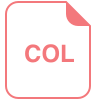
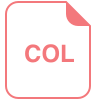
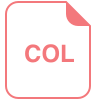
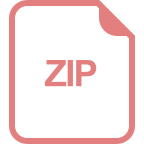
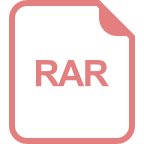