hex编码实现aes加密解密功能示例
时间: 2023-08-09 22:02:47 浏览: 79
hex编码实现AES加密解密功能示例可以按照以下步骤进行:
1. 导入所需的模块:
```python
import binascii
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
```
2. 定义AES加密和解密函数:
```python
def encrypt(plain_text, key):
cipher = AES.new(key, AES.MODE_EAX)
nonce = cipher.nonce
ciphertext, tag = cipher.encrypt_and_digest(plain_text.encode())
return binascii.hexlify(nonce + ciphertext + tag).decode()
def decrypt(cipher_text, key):
cipher_data = binascii.unhexlify(cipher_text)
nonce = cipher_data[:16]
ciphertext = cipher_data[16:-16]
tag = cipher_data[-16:]
cipher = AES.new(key, AES.MODE_EAX, nonce)
decrypted_text = cipher.decrypt_and_verify(ciphertext, tag)
return decrypted_text.decode()
```
3. 生成随机密钥:
```python
key = get_random_bytes(16)
```
4. 加密和解密示例数据:
```python
plain_text = "This is a sample plaintext."
cipher_text = encrypt(plain_text, key)
decrypted_text = decrypt(cipher_text, key)
```
完整的代码示例如下:
```python
import binascii
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
def encrypt(plain_text, key):
cipher = AES.new(key, AES.MODE_EAX)
nonce = cipher.nonce
ciphertext, tag = cipher.encrypt_and_digest(plain_text.encode())
return binascii.hexlify(nonce + ciphertext + tag).decode()
def decrypt(cipher_text, key):
cipher_data = binascii.unhexlify(cipher_text)
nonce = cipher_data[:16]
ciphertext = cipher_data[16:-16]
tag = cipher_data[-16:]
cipher = AES.new(key, AES.MODE_EAX, nonce)
decrypted_text = cipher.decrypt_and_verify(ciphertext, tag)
return decrypted_text.decode()
key = get_random_bytes(16)
plain_text = "This is a sample plaintext."
cipher_text = encrypt(plain_text, key)
decrypted_text = decrypt(cipher_text, key)
print("Plain text:", plain_text)
print("Encrypted text:", cipher_text)
print("Decrypted text:", decrypted_text)
```
这样,就可以使用hex编码实现AES加密解密功能了。
相关推荐
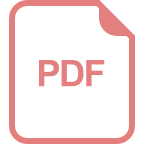
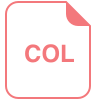
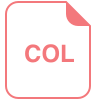
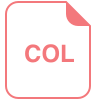
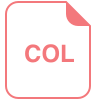










