pygame烟花代码设计思路
时间: 2024-05-26 15:09:25 浏览: 10
pygame烟花代码的设计思路大概如下:
1. 首先,需要导入pygame模块并初始化pygame。
2. 接下来,需要定义一个烟花类,该类包括以下属性:
- 烟花的位置
- 烟花的颜色
- 烟花的爆炸半径
- 烟花的速度
- 烟花的方向
3. 然后,需要定义一个粒子类,该类包括以下属性:
- 粒子的位置
- 粒子的颜色
- 粒子的速度
- 粒子的方向
4. 接下来,需要定义一个烟花爆炸函数,该函数接受一个烟花对象作为参数,然后在烟花位置创建一堆粒子。
5. 然后,在主循环中,需要检测用户输入(例如按下空格键),并在屏幕上创建一个新的烟花对象。
6. 最后,需要更新屏幕上的所有烟花和粒子,并在屏幕上绘制它们。
相关问题:
1. pygame是什么?
2. 什么是pygame的初始化?
3. pygame中的粒子类是什么?
4. 如何实现pygame烟花效果?
5. 如何在pygame中绘制对象?
相关问题
pygame测试代码
当然,Pygame是一个用于开发2D游戏的Python库。下面是一个简单的Pygame测试代码示例:
```python
import pygame
from pygame.locals import *
# 初始化Pygame
pygame.init()
# 设置窗口大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("Pygame测试")
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == QUIT:
running = False
# 渲染画面
screen.fill((255, 255, 255))
pygame.display.flip()
# 退出Pygame
pygame.quit()
```
这个代码创建了一个800x600的窗口,并在窗口中显示一个白色的背景。当点击窗口的关闭按钮时,游戏循环会结束,程序退出。
pygame斗地主代码
Pygame 是一个流行的 Python 游戏开发框架,可以用它来制作各种类型的游戏,包括斗地主。斗地主是一种扑克牌游戏,它通常由三个人玩。在这个游戏中,玩家需要在一定时间内获得最高分数,通过出牌和抢地主等方式来获取分数。下面是一个简单的 Pygame 斗地主代码示例:
```python
import pygame
# 初始化 Pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 加载背景图片
background_image = pygame.image.load("background.png")
# 设置游戏标题
pygame.display.set_caption("斗地主")
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 渲染背景图片
screen.blit(background_image, (0, 0))
# 刷新屏幕
pygame.display.flip()
# 退出 Pygame
pygame.quit()
```
这个示例代码中,我们使用 Pygame 初始化游戏、设置窗口、加载背景图片和设置游戏标题。然后进入游戏循环中,处理事件和渲染屏幕。在每次循环中,我们都会将背景图片渲染到屏幕上,并调用 `pygame.display.flip()` 函数来刷新屏幕。最后,当用户关闭游戏窗口时,我们会退出 Pygame。
如果你需要更详细的代码示例,可以在网上搜索相关资源。
相关推荐
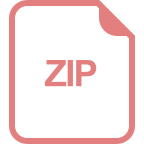
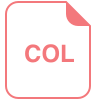
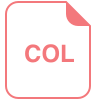
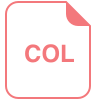
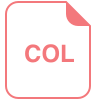
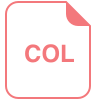







