sqlsugar操作oracle
时间: 2024-06-04 12:04:30 浏览: 170
SQLSugar是一个ORM框架,可以简化对关系型数据库的操作。它提供了对Oracle、SQL Server、MySQL、SQLite等多种数据库的支持。下面介绍一下SQLSugar操作Oracle的方法:
1. 引用Oracle.DataAcess.dll
需要在项目中引用Oracle.DataAcess.dll,可以在NuGet上下载。
2. 配置连接字符串
使用SQLSugar连接Oracle数据库时,需要配置连接字符串。可以在Web.config或者App.config中添加以下代码:
```
<connectionStrings>
<add name="OracleConnectionString" connectionString="Data Source=ORACLE;User ID=USERNAME;Password=PASSWORD;"/>
</connectionStrings>
```
这里需要替换ORACLE、USERNAME和PASSWORD为实际的值。
3. 配置SqlSugarClient
在使用SqlSugar之前,需要先配置SqlSugarClient。代码如下:
```
using SqlSugar;
public class OracleDbContext
{
public static SqlSugarClient GetInstance()
{
var db = new SqlSugarClient(new ConnectionConfig()
{
ConnectionString = ConfigurationManager.ConnectionStrings["OracleConnectionString"].ConnectionString,
DbType = DbType.Oracle,
IsAutoCloseConnection = true,
InitKeyType = InitKeyType.Attribute,
ConfigureExternalServices = new ConfigureExternalServices()
{
EntityService = (property, column) =>
{
if (property.Name.Equals("Id"))
return new EntityColumnInfo() { ColumnName = "ID", IsPrimarykey = true };
else
return new EntityColumnInfo() { ColumnName = column.DbColumnName };
}
}
});
return db;
}
}
```
4. 使用SqlSugar进行操作
有了SqlSugarClient之后,就可以进行数据库操作了。以下是一些常用的操作方法:
- 查询所有记录
```
var list = db.Queryable<Student>().ToList();
```
- 根据条件查询
```
var list = db.Queryable<Student>().Where(s => s.Name == "Tom").ToList();
```
- 分页查询
```
var list = db.Queryable<Student>().Where(s => s.Age > 20).OrderBy(s => s.Id).ToPageList(2, 10);
```
- 插入记录
```
var student = new Student() { Name = "Tom", Age = 25 };
db.Insertable(student).ExecuteCommand();
```
- 更新记录
```
db.Updateable<Student>().SetColumns(s => new Student() { Age = 26 }).Where(s => s.Name == "Tom").ExecuteCommand();
```
- 删除记录
```
db.Deleteable<Student>().Where(s => s.Id == 1).ExecuteCommand();
```
阅读全文
相关推荐
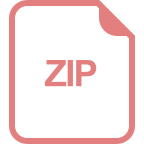





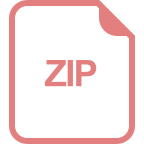









