c语言冒泡排序返回新数组
时间: 2024-12-28 12:41:42 浏览: 0
在C语言中,当你想要对一个数组进行冒泡排序并且不想改变原始数组,通常会创建一个新的数组来存储排序后的结果,因为原地冒泡排序(直接修改输入数组)会改变元素位置。下面是一个简单的冒泡排序算法示例,它返回一个新的已排序数组:
```c
#include <stdio.h>
#include <stdlib.h>
// 冒泡排序函数,接受数组、长度和结果数组指针作为参数
void bubbleSort(int arr[], int n, int result[], int size) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// 交换元素
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
// 将已排序的元素复制到结果数组
result[size - 1 - j] = arr[j]; // 注意大小减一,从后向前填充
}
}
}
}
int main() {
int input[] = {5, 2, 8, 1, 9};
int size = sizeof(input) / sizeof(input[0]);
// 初始化一个足够大的结果数组
int* sortedArray = malloc(size * sizeof(int));
bubbleSort(input, size, sortedArray, size);
printf("Sorted array is: ");
for (int i = 0; i < size; i++) {
printf("%d ", sortedArray[i]);
}
free(sortedArray); // 别忘了释放内存
return 0;
}
```
在这个例子中,`bubbleSort`函数将原始数组`input`按升序排序,并将结果保存在`result`数组中。注意,由于需要预先分配空间给`result`,因此需要知道输入数组的大小。程序最后会打印出排序后的数组并释放动态分配的内存。
阅读全文
相关推荐
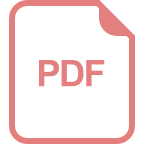
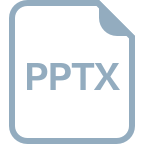
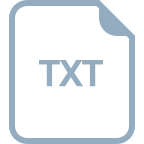
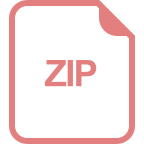
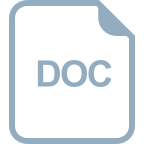
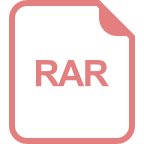
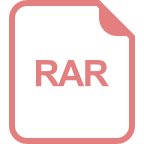
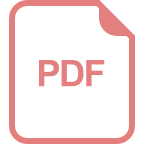
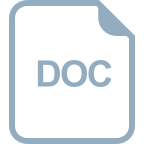
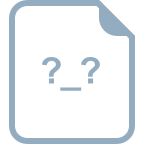
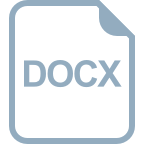
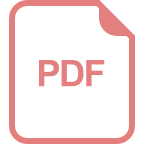
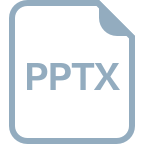
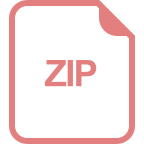
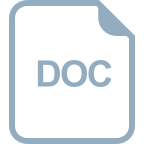
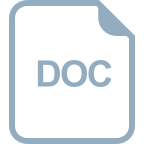