数据结构——用c语言描述(第3版)课后答案
时间: 2023-10-25 17:04:46 浏览: 431
《数据结构——用C语言描述(第3版)》课后答案的描述使用C语言来实现各种数据结构和算法。以下是对几个常见数据结构的描述和相关代码示例。
1. 数组(Array):数组是一种线性数据结构,用于存储相同类型的元素。C语言中使用数组可以快速访问和修改元素。示例代码如下:
```c
#include <stdio.h>
int main() {
int arr[5] = {1, 2, 3, 4, 5};
for(int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
return 0;
}
```
2. 链表(Linked List):链表是一种动态数据结构,通过节点之间的指针链接来存储数据。C语言中可以使用结构体和指针来实现链表。示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void printList(struct Node* head) {
struct Node* current = head;
while(current != NULL) {
printf("%d ", current->data);
current = current->next;
}
}
int main() {
struct Node* head = NULL;
struct Node* second = NULL;
struct Node* third = NULL;
head = (struct Node*) malloc(sizeof(struct Node));
second = (struct Node*) malloc(sizeof(struct Node));
third = (struct Node*) malloc(sizeof(struct Node));
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
printList(head);
return 0;
}
```
3. 栈(Stack):栈是一种后进先出(LIFO)的数据结构,在C语言中可以使用数组来实现。示例代码如下:
```c
#include <stdio.h>
#define MAX_SIZE 100
int stack[MAX_SIZE];
int top = -1;
void push(int item) {
if(top == MAX_SIZE - 1) {
printf("Stack Overflow\n");
} else {
stack[++top] = item;
}
}
int pop() {
if(top == -1) {
printf("Stack Underflow\n");
return -1;
} else {
return stack[top--];
}
}
void printStack() {
for(int i = top; i >= 0; i--) {
printf("%d ", stack[i]);
}
}
int main() {
push(1);
push(2);
push(3);
printf("Popped element: %d\n", pop());
printStack();
return 0;
}
```
这些示例代码展示了如何使用C语言描述《数据结构——用C语言描述(第3版)》中介绍的数据结构。读者可以根据书中提供的习题进行编程练习,进一步巩固数据结构和算法的相关知识。
相关推荐
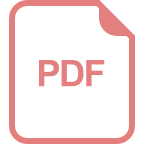
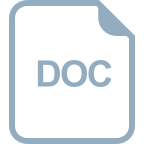
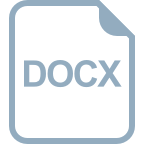














