编写一个程序 接受用户输入一行英文句子(假设该句子仅由英文单词及空格构成,不包括逗号等符号),统计并输出该句子包含的单词个数及单词的平均长度。
时间: 2024-10-28 19:08:37 浏览: 40
编写这样一个程序可以分为以下几个步骤:
1. **获取用户输入**:首先,你需要创建一个函数来接收用户的输入,例如在Python中可以使用`input()`函数。
```python
def get_user_input():
sentence = input("请输入一行英文句子:")
return sentence.strip()
```
2. **分割单词**:然后,我们需要将句子按空格分割成单词列表。Python的`split()`函数非常适合这个任务。
```python
def split_words(sentence):
words = sentence.split(' ')
return words
```
3. **计算单词个数**:遍历列表,计算单词的数量。
```python
def count_words(words):
word_count = len(words)
return word_count
```
4. **计算平均单词长度**:对于每个单词,获取其长度并累加,最后除以单词总数得到平均长度。
```python
def average_word_length(words):
total_length = sum(len(word) for word in words)
if word_count > 0:
average_length = total_length / word_count
return average_length
else:
return 0 # 防止除零错误,当没有单词时返回0
```
5. **整合所有功能**:将上述部分组合起来,并打印结果。
```python
def main():
sentence = get_user_input()
words = split_words(sentence)
word_count = count_words(words)
avg_length = average_word_length(words)
print(f"句子中有 {word_count} 个单词,平均单词长度为 {avg_length:.2f} 字符.")
if __name__ == "__main__":
main()
```
阅读全文
相关推荐
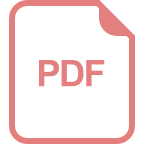
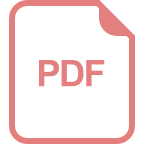
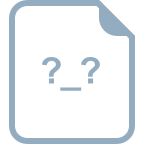






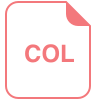
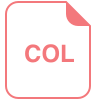
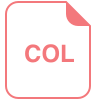
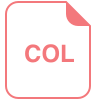
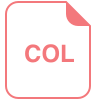
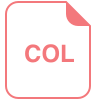
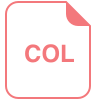

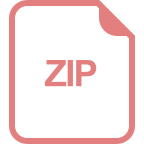
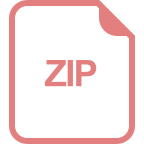